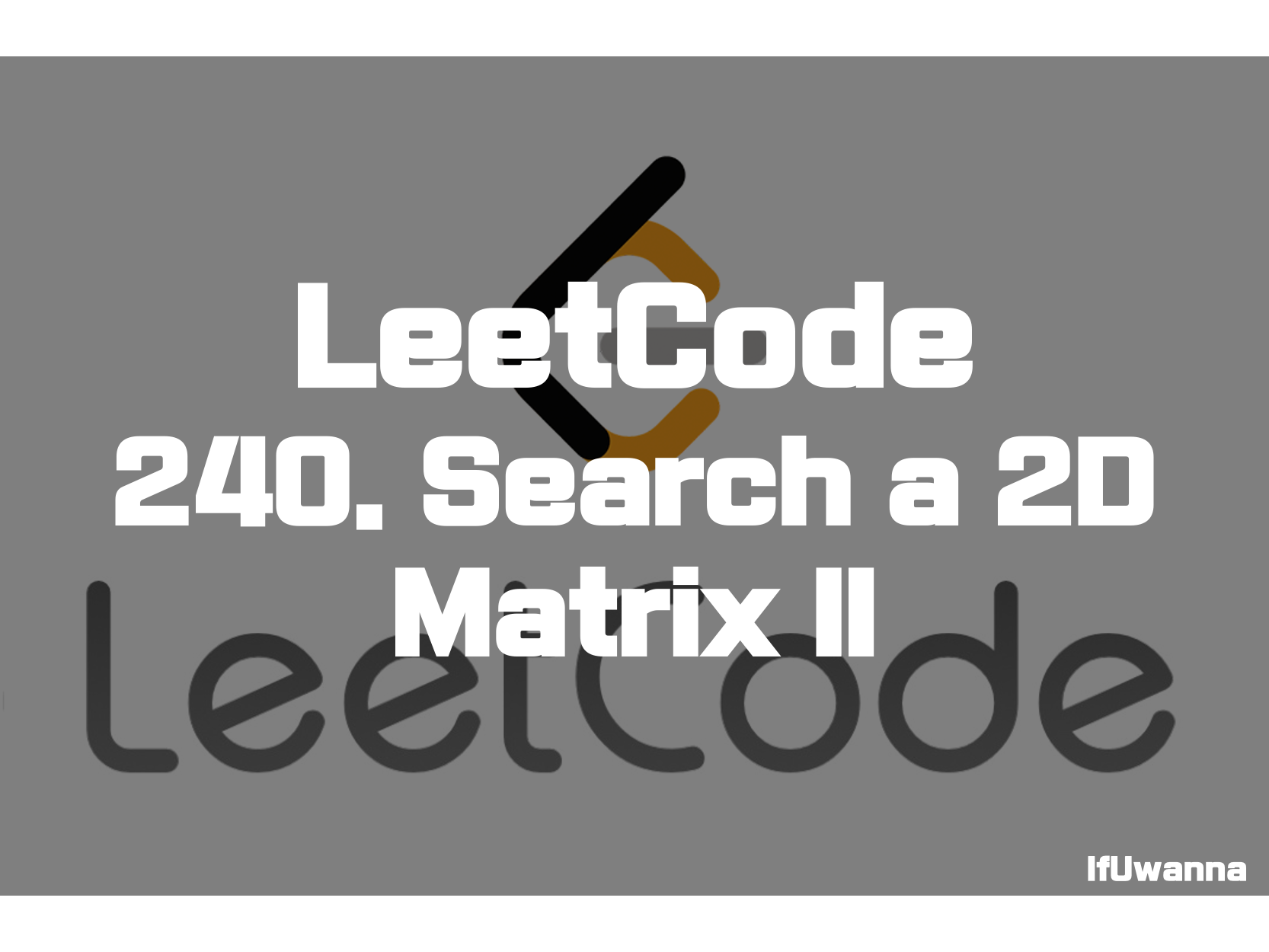
Description
각 행,열이 오름차순의 값을 가지고 있는 배열이 주어졌을때 target의 존재 여부를 반환하는 문제입니다.
Write an efficient algorithm that searches for a value target in an m x n integer matrix matrix. This matrix has the following properties:
- Integers in each row are sorted in ascending from left to right.
- Integers in each column are sorted in ascending from top to bottom.
Example 1:
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 5
Output: true
Example 2:
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 20
Output: false
Constraints:
- m == matrix.length
- n == matrix[i].length
- 1 <= n, m <= 300
- -10^9 <= matrix[i][j] <= 10^9
- All the integers in each row are sorted in ascending order.
- All the integers in each column are sorted in ascending order.
- -10^9 <= target <= 10^9
Solution 1. Binary Search
public boolean searchMatrix(int[][] matrix, int target) {
int m = matrix.length;
int n = matrix[0].length;
if(matrix == null || m < 1 || n <1) {
return false;
}
int r = 0;
int c = n-1;
while(r >= 0 && r <= m-1 && c >=0 && c<=n-1){
if(target == matrix[r][c]) {
return true;
} else if(target < matrix[r][c]) {
c--;
} else if(target > matrix[r][c]) {
r++;
}
}
return false;
}
현재 위치를 오른쪽 상단 모서리로 초기화하여 검색을 시작합니다. 정렬되어 있기에 오른쪽 맨 끝이 가장 큰값이므로 해당 값보다 큰값이면 아래 Row를 탐색하고 작을 경우 앞쪽 Column을 탐색합니다.
Reference
Search a 2D Matrix II - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 1249. Minimum Remove to Make Valid Parentheses -문제풀이 (0) | 2022.03.15 |
---|---|
[LeetCode] 71. Simplify Path - 문제풀이 (0) | 2022.03.15 |
[LeetCode] 59. Spiral Matrix II - 문제풀이 (0) | 2022.03.12 |
[LeetCode] 48. Rotate Image - 문제풀이 (0) | 2022.03.12 |
[LeetCode] 119. Pascal's Triangle II - 문제풀이 (0) | 2022.03.12 |