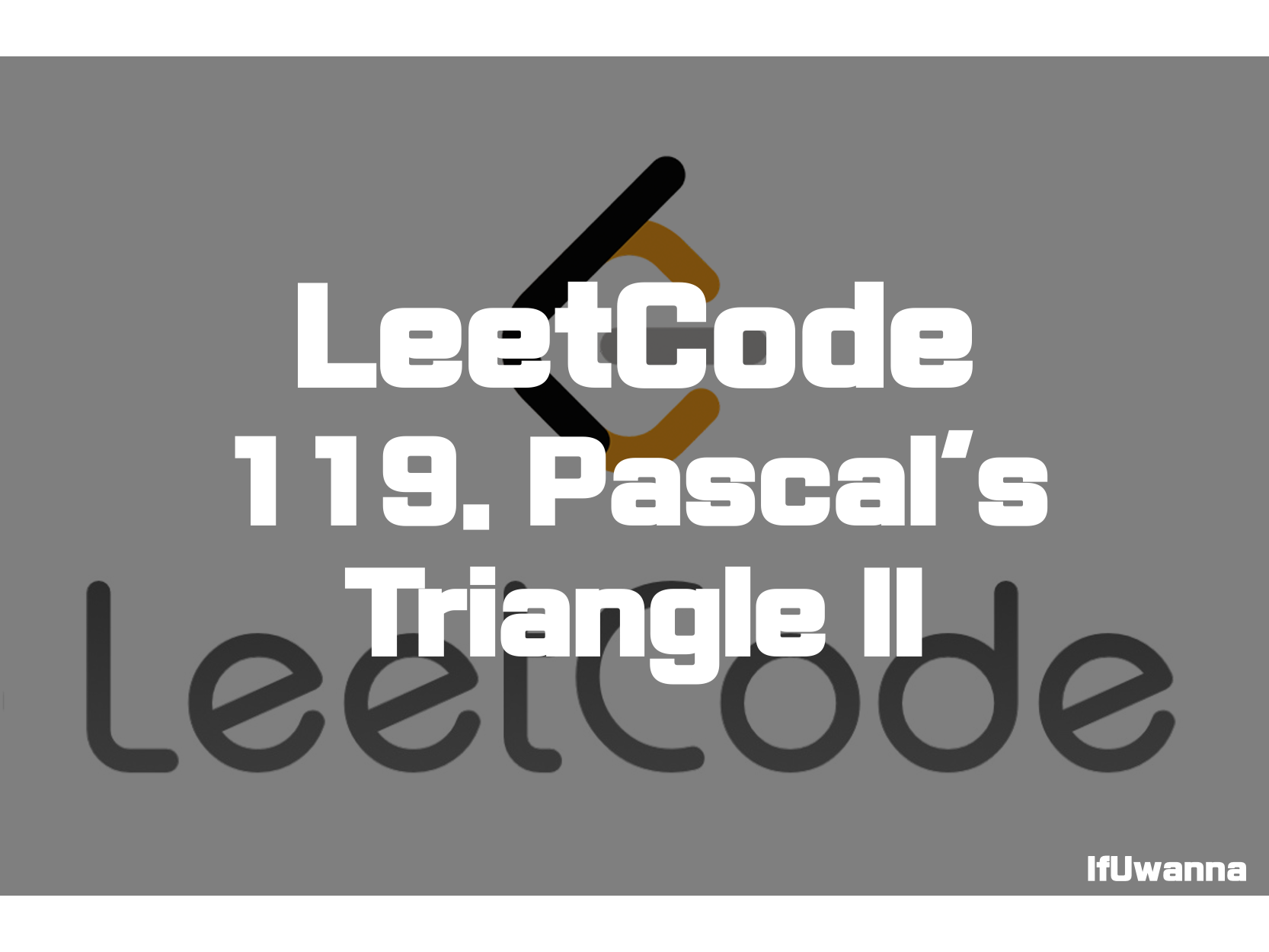
Description
파스칼의 삼각형을 계산하여 주어진 rowIndex의 값들을 반환하는 문제입니다.
Given an integer rowIndex, return the rowIndexth (0-indexed) row of the Pascal's triangle.
In Pascal's triangle, each number is the sum of the two numbers directly above it as shown:
Example 1:
Input: rowIndex = 3
Output: [1,3,3,1]
Example 2:
Input: rowIndex = 0
Output: [1]
Example 3:
Input: rowIndex = 1
Output: [1,1]
Constraints
- 0 <= rowIndex <= 33
Solution 1. Dynamic Programming
public List<Integer> getRow(int rowIndex) {
List<List<Integer>> triangle = new ArrayList<>();
for (int i = 0; i <= rowIndex; i++) {
List<Integer> row = new ArrayList<>();
for (int j = 0; j <= i; j++) {
if(j==0 || j==i){
row.add(1);
}else {
row.add(triangle.get(i-1).get(j-1) + triangle.get(i-1).get(j));
}
}
triangle.add(row);
}
return triangle.get(triangle.size()-1);
}
제일 첫번째 ROW부터 탑다운 방식으로 값을 채워나가며 주어진 마지막 rowIndex의 값을 반환해줍니다.
Reference
Pascal's Triangle II - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 59. Spiral Matrix II - 문제풀이 (0) | 2022.03.12 |
---|---|
[LeetCode] 48. Rotate Image - 문제풀이 (0) | 2022.03.12 |
[LeetCode] 61. Rotate List - 문제풀이 (0) | 2022.03.11 |
[LeetCode] 2. Add Two Numbers - 문제풀이 (0) | 2022.03.10 |
[LeetCode] 82. Remove Duplicates from Sorted List II - 문제풀이 (0) | 2022.03.10 |