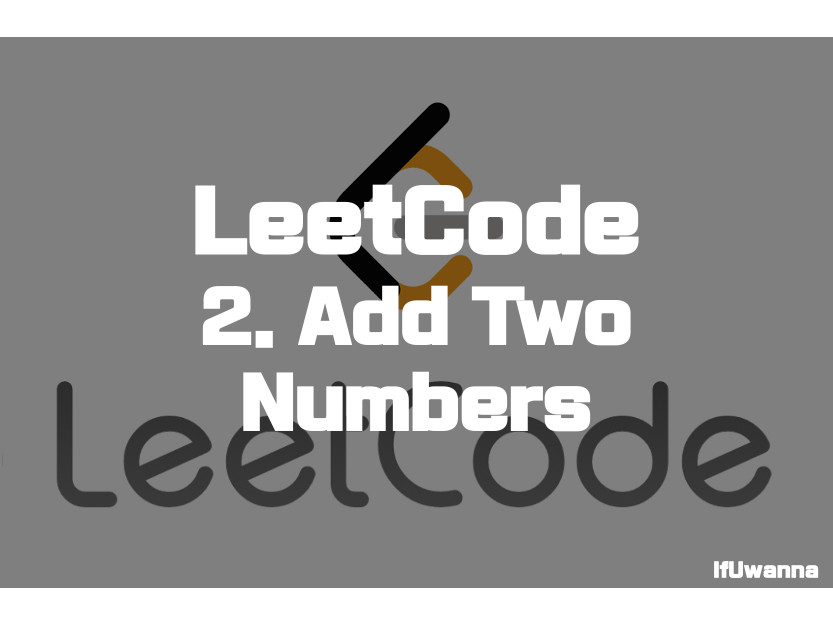
Description
주어진 두 링크드 리스트의 역순으로 읽은 숫자의 합을 역순으로 반환하는 문제입니다.
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1:
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0]
Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
Constraints:
- The number of nodes in each linked list is in the range [1, 100].
- 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
Solution 1. Math
public ListNode addTwoNumbers(ListNode l1, ListNode l2) {
ListNode dHead = new ListNode(0);
ListNode p = l1;
ListNode q = l2;
ListNode cur = dHead;
int carry = 0;
while (p != null || q != null) {
int x = (p != null) ? p.val : 0;
int y = (q != null) ? q.val : 0;
int sum = carry + x + y;
carry = sum / 10;
cur.next = new ListNode(sum % 10);
cur = cur.next;
if (p != null) p = p.next;
if (q != null) q = q.next;
}
if (carry > 0) {
cur.next = new ListNode(carry);
}
return dHead.next;
}
우리가 두 숫자의 합을 뒤에서부터 계산해 나가는 것 처럼 역순으로 계산하면서 올림값이 발생하면 다음 노드에 하나 추가로 더해줍니다.
Reference
Add Two Numbers - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 119. Pascal's Triangle II - 문제풀이 (0) | 2022.03.12 |
---|---|
[LeetCode] 61. Rotate List - 문제풀이 (0) | 2022.03.11 |
[LeetCode] 82. Remove Duplicates from Sorted List II - 문제풀이 (0) | 2022.03.10 |
[LeetCode] 706. Design HashMap - 문제풀이 (0) | 2022.03.08 |
[LeetCode] 56. Merge Intervals - 문제풀이 (0) | 2022.03.08 |