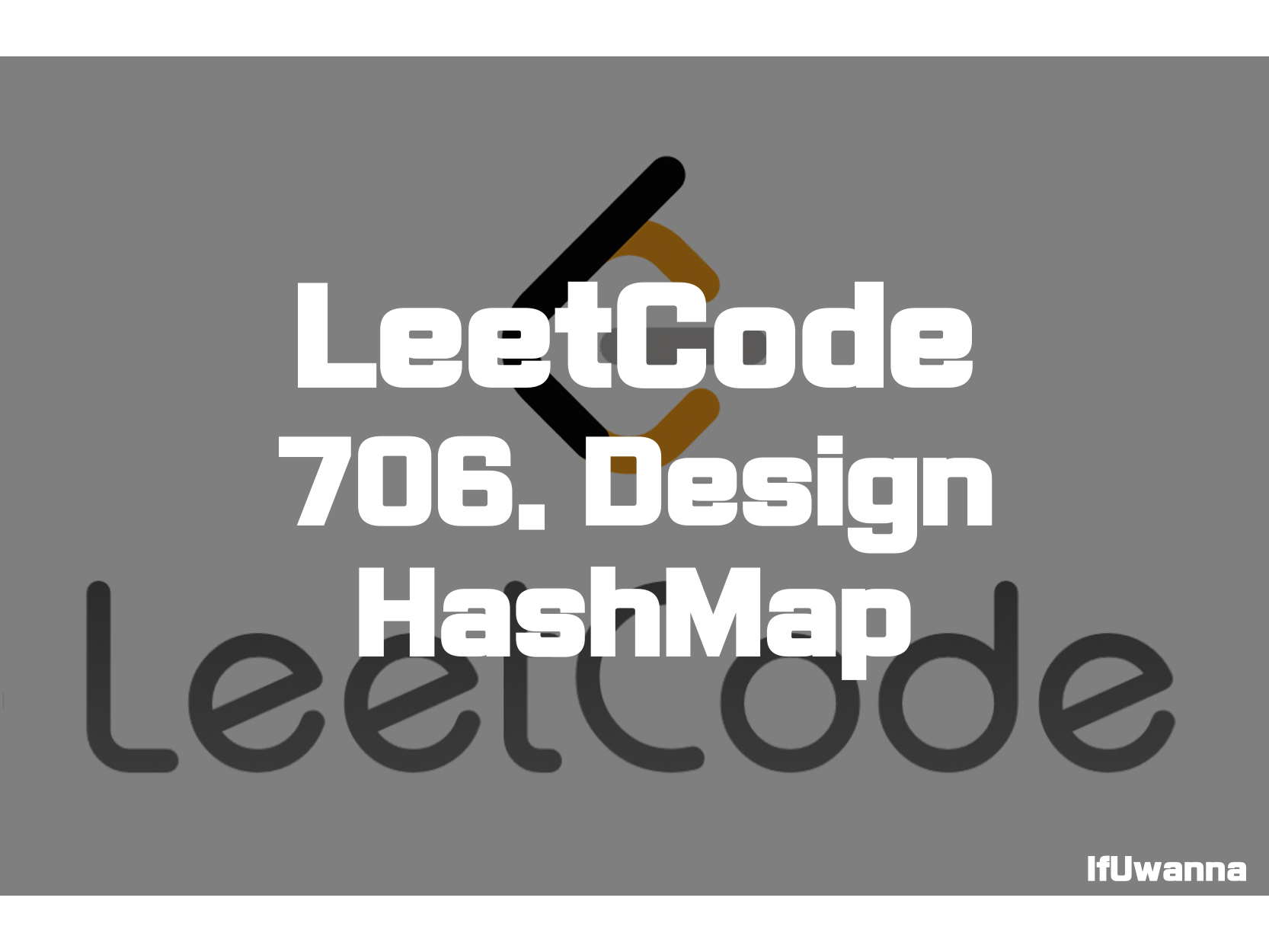
Description
해시테이블 라이브러리를 사용하지 않고 HashMap을 구현하는 문제입니다.
Design a HashMap without using any built-in hash table libraries.
Implement the MyHashMap class:
- MyHashMap() initializes the object with an empty map.
- void put(int key, int value) inserts a (key, value) pair into the HashMap. If the key already exists in the map, update the corresponding value.
- int get(int key) returns the value to which the specified key is mapped, or 1 if this map contains no mapping for the key.
- void remove(key) removes the key and its corresponding value if the map contains the mapping for the key.
Example 1:
Input
["MyHashMap", "put", "put", "get", "get", "put", "get", "remove", "get"]
[[], [1, 1], [2, 2], [1], [3], [2, 1], [2], [2], [2]]
Output
[null, null, null, 1, -1, null, 1, null, -1]
Explanation
MyHashMap myHashMap = new MyHashMap();
myHashMap.put(1, 1); // The map is now [[1,1]]
myHashMap.put(2, 2); // The map is now [[1,1], [2,2]]
myHashMap.get(1); // return 1, The map is now [[1,1], [2,2]]
myHashMap.get(3); // return -1 (i.e., not found), The map is now [[1,1], [2,2]]
myHashMap.put(2, 1); // The map is now [[1,1], [2,1]] (i.e., update the existing value)
myHashMap.get(2); // return 1, The map is now [[1,1], [2,1]]
myHashMap.remove(2); // remove the mapping for 2, The map is now [[1,1]]
myHashMap.get(2); // return -1 (i.e., not found), The map is now [[1,1]]
Constraints:
- 0 <= key, value <= 10^6
- At most 10^4 calls will be made to put, get, and remove.
Solution 1. Using Array
public class MyHashMap {
int[] map;
public MyHashMap() {
map = new int[1000001];
Arrays.fill(map,-1);
}
public void put(int key, int value) {
map[key] = value;
}
public int get(int key) {
return map[key];
}
public void remove(int key) {
map[key] = -1;
}
}
index를 키로 가지고 있는 배열을 저장공간으로 사용하여 hashmap을 구현할 수 있습니다. 0~100000 값이 들어 올 수 있으므로 인덱스를 키로 사용하기위해 100001 공간을 사용하는 배열을 선언하고 값역시 0~100000까지 들어 올 수 있으므로 초기값을 -1로 채워줍니다.
Reference
Design HashMap - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 2. Add Two Numbers - 문제풀이 (0) | 2022.03.10 |
---|---|
[LeetCode] 82. Remove Duplicates from Sorted List II - 문제풀이 (0) | 2022.03.10 |
[LeetCode] 56. Merge Intervals - 문제풀이 (0) | 2022.03.08 |
[LeetCode] 75. Sort Colors - 문제풀이 (0) | 2022.03.08 |
[LeetCode] 15. 3Sum - 문제풀이 (0) | 2022.03.07 |