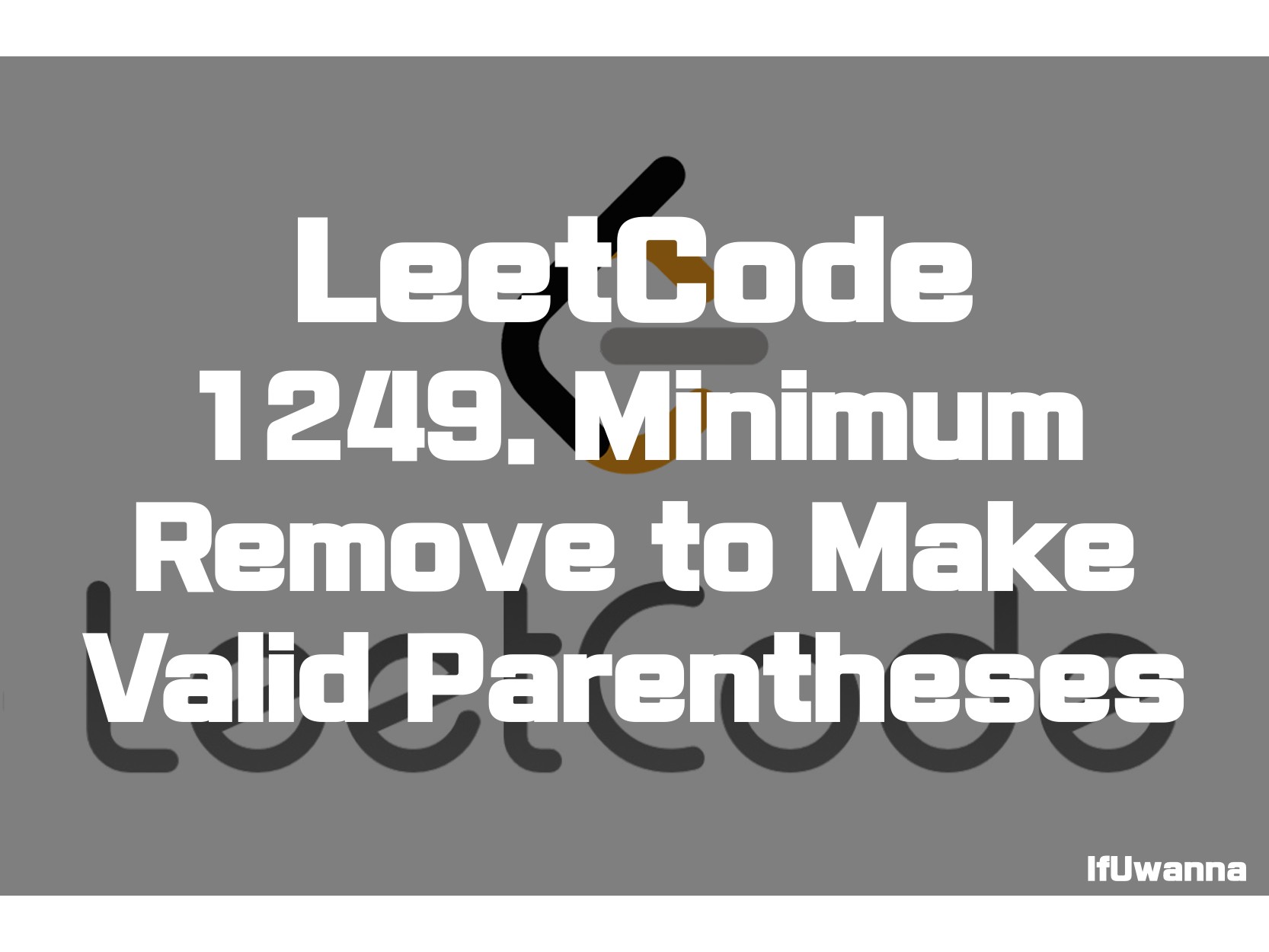
Description
소괄호가 유효하도록 문자열을 수정하여 반환하는 문제입니다.
Given a string s of '(' , ')' and lowercase English characters.
Your task is to remove the minimum number of parentheses ( '(' or ')', in any positions ) so that the resulting parentheses string is valid and return any valid string.
Formally, a parentheses string is valid if and only if:
- It is the empty string, contains only lowercase characters, or
- It can be written as AB (A concatenated with B), where A and B are valid strings, or
- It can be written as (A), where A is a valid string.
Example 1:
Input: s = "lee(t(c)o)de)"
Output: "lee(t(c)o)de"
Explanation: "lee(t(co)de)" , "lee(t(c)ode)" would also be accepted.
Example 2:
Input: s = "a)b(c)d"
Output: "ab(c)d"
Example 3:
Input: s = "))(("
Output: ""
Explanation: An empty string is also valid.
Constraints:
- 1 <= s.length <= 105
- s[i] is either'(' , ')', or lowercase English letter.
Solution 1. Iteration
public String minRemoveToMakeValid(String s) {
int validCnt = 0;
StringBuilder sb = new StringBuilder();
for(char c : s.toCharArray()){
if(c=='('){
sb.append(c);
validCnt++;
}else if(c ==')' && validCnt > 0){
sb.append(c);
validCnt--;
}else if(Character.isLetter(c)){
sb.append(c);
}
}
for (int i = 0; i < validCnt ; i++) {
sb.deleteCharAt(sb.lastIndexOf("("));
}
return sb.toString();
}
문자열을 반복하며 열린 괄호가 나올 경우 유효한 닫힌괄호개수를 체크하고 유효할 경우에만 결과에 추가해줍니다. 괄호가 열리고 끝나지 않는 케이스는 모든 문자열을 반복 한 뒤 validCnt가 남아있는 케이스 이므로 뒤에서부터 열린괄호를 제거 해줍니다.
Reference
Minimum Remove to Make Valid Parentheses - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 856. Score of Parentheses - 문제풀이 (0) | 2022.03.17 |
---|---|
[LeetCode] 946. Validate Stack Sequences - 문제풀이 (0) | 2022.03.16 |
[LeetCode] 71. Simplify Path - 문제풀이 (0) | 2022.03.15 |
[LeetCode] 240. Search a 2D Matrix II - 문제풀이 (0) | 2022.03.12 |
[LeetCode] 59. Spiral Matrix II - 문제풀이 (0) | 2022.03.12 |