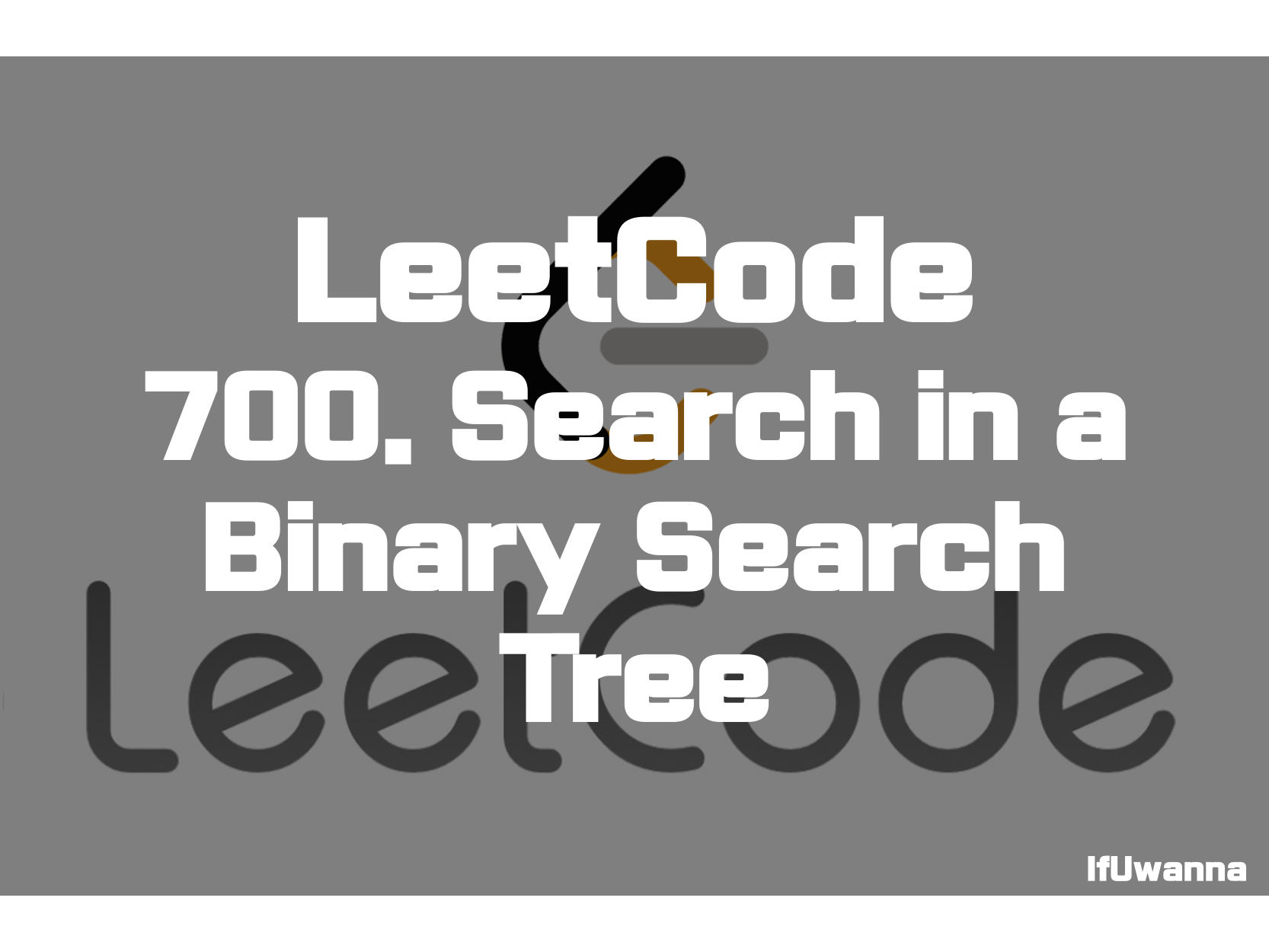
Description
주어진 이진검색트리(BST)에서 val와 같은 값을 가지는 노드를 반환하는 문제입니다.
You are given the root of a binary search tree (BST) and an integer val.
Find the node in the BST that the node's value equals val and return the subtree rooted with that node. If such a node does not exist, return null.
Example 1:
Input: root = [4,2,7,1,3], val = 2
Output: [2,1,3]
Example 2:
Input: root = [4,2,7,1,3], val = 5
Output: []
Constraints:
- The number of nodes in the tree is in the range [1, 5000].
- 1 <= Node.val <= 10^7
- root is a binary search tree.
- 1 <= val <= 10^7
Solution 1. DFS - Recursion(BST)
public TreeNode searchBST(TreeNode root, int val) {
//1. Recursion - DFS (Binary Search Tree)
if(root == null) return null;
if(root.val == val) return root;
return root.val > val? searchBST(root.left,val) : searchBST(root.right,val);
}
깊이우선탐색(DFS)을 이용하여 재귀호출을 통해 일치하는 노드를 찾아갑니다.
Solution2. DFS - Recursion(BT)
public TreeNode searchBST(TreeNode root, int val) {
//2. Recursion - DFS (Normal Binary Tree)
if(root == null) return null;
if(root.val == val) return root;
TreeNode left = searchBST(root.left,val);
if(left != null){
return left;
}else{
return searchBST(root.right,val);
}
}
값이 root기준으로 정렬되어 있지 않는 일반 이진 트리일 경우 깊이우선탐색(DFS)을 이용하여 재귀호출을 통해 일치하는 노드를 찾아가는 코드입니다.
Solution 3. Iteration(BST)
public TreeNode searchBST(TreeNode root, int val) {
//3. Iteration - BFS (Binary Search Tree)
if(root == null) return null;
while (root != null && root.val != val) {
root = root.val < val ? root.right : root.left;
}
return root;
}
반복을 통해 현재 루트 기준으로 기준값이 작을 경우 왼쪽노드를, 아닌 경우 오른쪽 노드로 이동하여 탐색해 나갑니다.
Solution4. BFS - Iteration(BT)
public TreeNode searchBST4(TreeNode root, int val) {
//4. Iteration - BFS (Normal Binary Tree)
if(root == null) return null;
Queue<TreeNode> q = new LinkedList<>();
q.offer(root);
while(!q.isEmpty()){
TreeNode cur = q.poll();
if(cur.val == val) return cur;
if(cur.left != null) q.offer(cur.left);
if(cur.right != null) q.offer(cur.right);
}
return null;
}
값이 root기준으로 정렬되어 있지 않는 일반 이진 트리일 경우 너비우선탐색(DFS)을 이용하여 반복적으로 큐에 노드를 넣고 모든 노드를 검사해가며 찾아갑니다.
Reference
Search in a Binary Search Tree - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[Leetcode] 98. Validate Binary Search Tree - 문제풀이 (0) | 2022.02.14 |
---|---|
[LeetCode] 701. Insert into a Binary Search Tree - 문제풀이 (0) | 2022.02.14 |
[LeetCode ] 112. Path Sum - 문제풀이 (0) | 2022.02.14 |
[LeetCode] 226. Invert Binary Tree - 문제풀이 (0) | 2022.02.14 |
[LeetCode] 101. Symmetric Tree - 문제풀이 (0) | 2022.02.14 |