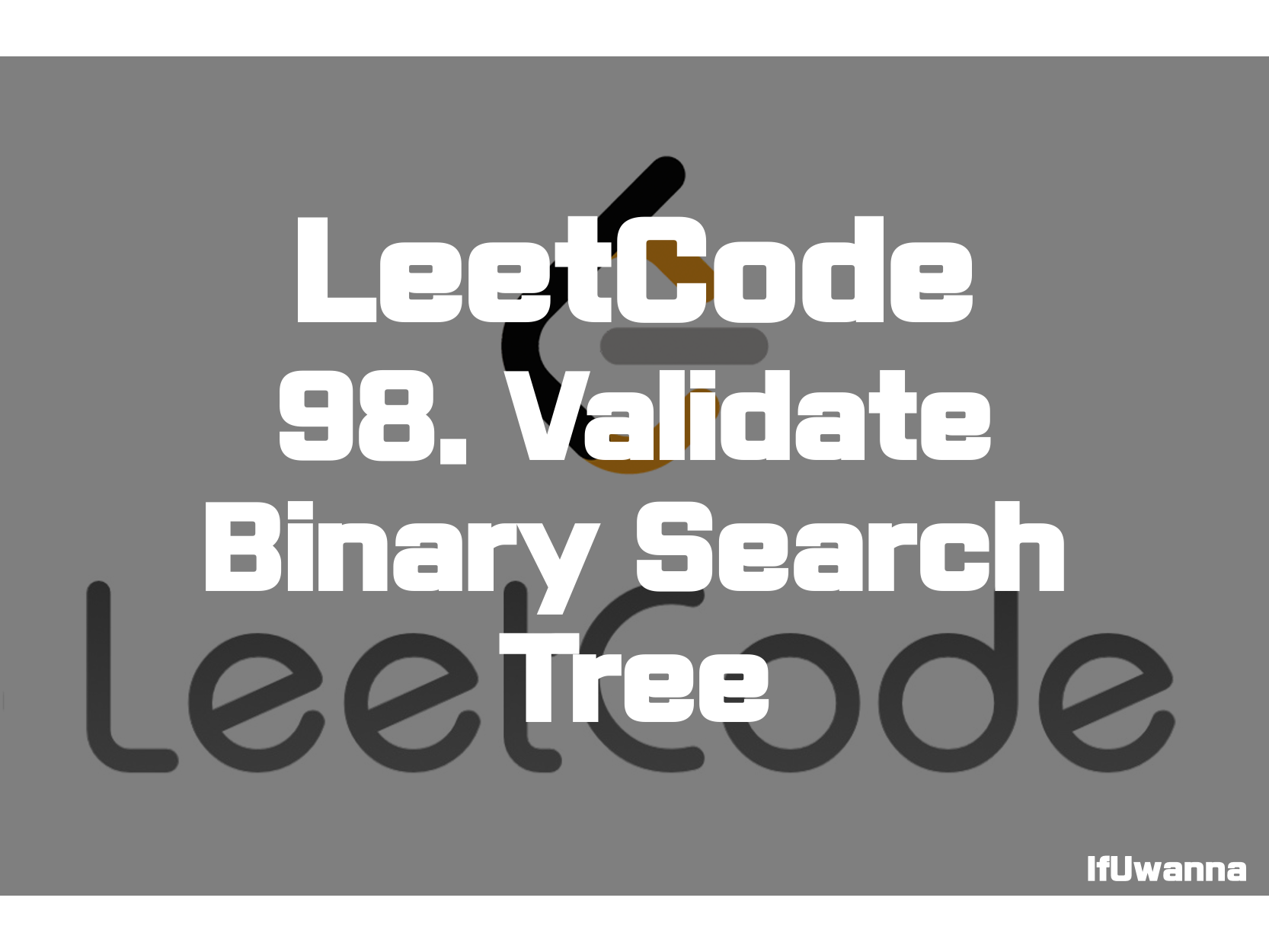
Description
주어진 이진트리가 유효한 이진탐색트리인지 여부를 반환하는 문제입니다.
Given the root of a binary tree, determine if it is a valid binary search tree (BST).
A valid BST is defined as follows:
- The left subtree of a node contains only nodes with keys less than the node's key.
- The right subtree of a node contains only nodes with keys greater than the node's key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
Input: root = [2,1,3]
Output: true
Example 2:
Input: root = [5,1,4,null,null,3,6]
Output: false
Explanation: The root node's value is 5 but its right child's value is 4.
Constraints:
- The number of nodes in the tree is in the range [1, 10^4].
- -2^31 <= Node.val <= 2^31 - 1
Solution 1. Recursion(재귀)
public boolean isValidBST(TreeNode root, Integer min, Integer max) {
//1. Recursion - DFS
if(root == null) return true;
if(min != null && root.val <= min) return false;
if(max != null && root.val >= max) return false;
return isValidBST(root.left, min, root.val) && isValidBST(root.right, root.val, max);
}
깊이우선탐색 재귀호출을 통하여 노드를 탐색해 나가며 유효성을 체크합니다. 왼쪽 노드는 root노드보다 클 수 없고 오른쪽노드는 root노드보다 작을 수 없기때문에 최소,최대값을 유지해나가며 재귀호출을 실행합니다.
Reference
Validate Binary Search Tree - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 235. Lowest Common Ancestor of a Binary Search Tree - 문제풀이 (0) | 2022.02.14 |
---|---|
[LeetCode] 653. Two Sum IV - Input is a BST - 문제풀이 (0) | 2022.02.14 |
[LeetCode] 701. Insert into a Binary Search Tree - 문제풀이 (0) | 2022.02.14 |
[LeetCode] 700. Search in a Binary Search Tree - 문제풀이 (0) | 2022.02.14 |
[LeetCode ] 112. Path Sum - 문제풀이 (0) | 2022.02.14 |