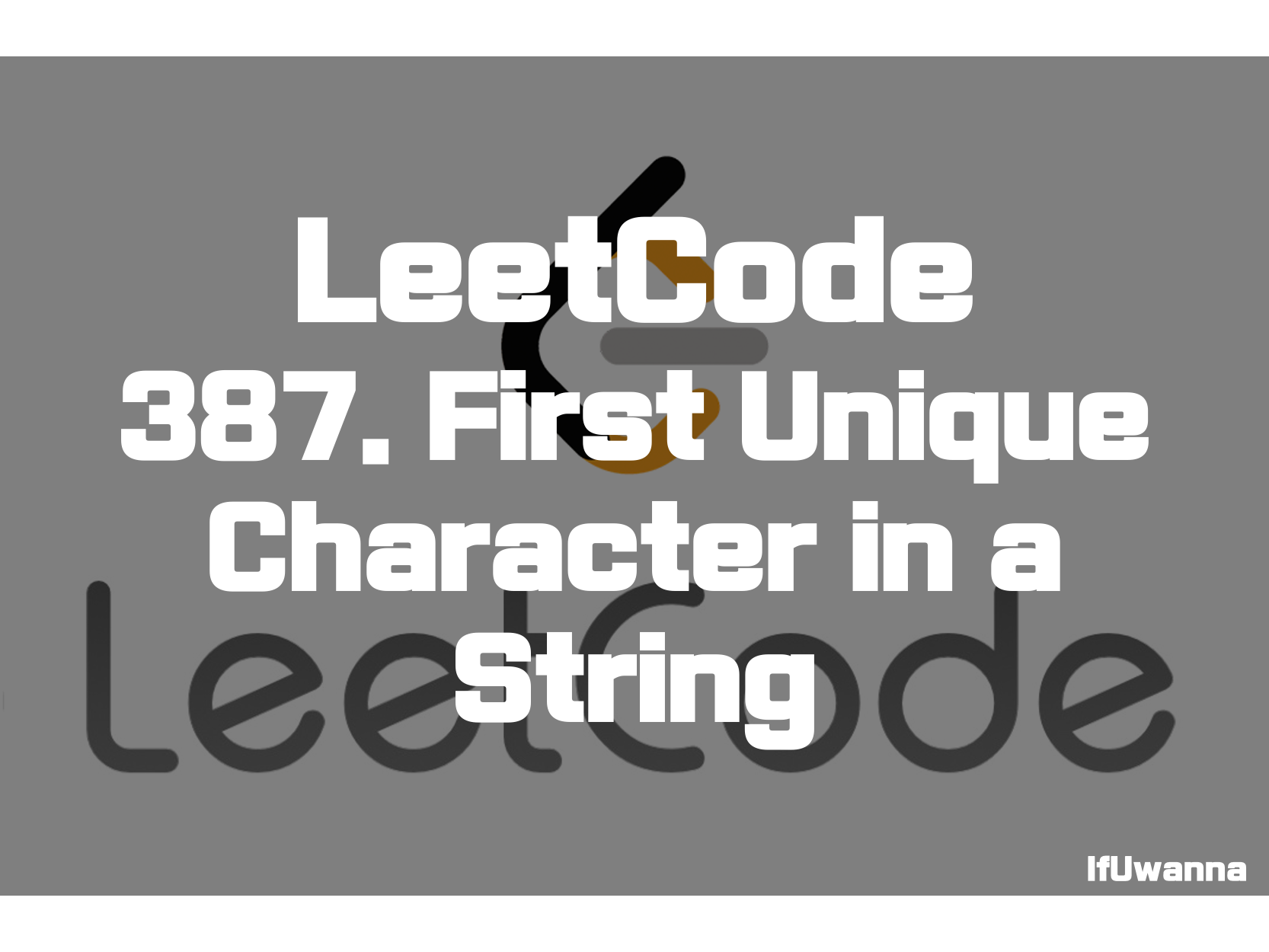
Description
주어진 문자열 s에서 겹치지 않는 첫번째 문자를 찾아내는 문제입니다.
Given a string s, find the first non-repeating character in it and return its index. If it does not exist, return -1.
Example 1:
Input: s = "leetcode"
Output: 0
Example 2:
Input: s = "loveleetcode"
Output: 2
Example 3:
Input: s = "aabb"
Output: -1
Constraints:
- 1 <= s.length <= 105
- s consists of only lowercase English letters.
Solution 1. IndexOf()
public int firstUniqChar(String s) {
//1. indexOf
boolean[] isChecked = new boolean[26];
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
int idx = c - 'a';
if (isChecked[idx]) continue;
if (!isChecked[idx] && s.indexOf(c, i + 1) < 0) {
return i;
}
isChecked[idx] = true;
}
return -1;
}
IndexOf() 메서드를 이용하여 다음 문자열에 속하는게 있는지 확인하고 이미 확인한 문자는 스킵해가며 끝까지 진행합니다.
Solution 2.
public int firstUniqChar(String s) {
//2. hash table
int[] map = new int[26];
for (int i = 0; i < s.length(); i++) {
map[s.charAt(i)-'a']++;
}
for (int i = 0; i < s.length(); i++) {
if(map[s.charAt(i)-'a'] == 1){
return i;
}
}
return -1;
}
각 문자열을 index로 하는 배열을 이용해 각 알파벳별 사용된 count를 기록하고 한번 만 사용된 첫번째 인덱스를 반환 합니다.
Reference
First Unique Character in a String - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 520. Detect Capital - 문제풀이 (0) | 2022.01.25 |
---|---|
[LeetCode] 383. Ransom Note - 문제풀이 (0) | 2022.01.24 |
[LeetCode] 134. Gas Station - 문제풀이 (0) | 2022.01.23 |
[LeetCode] 2148. Count Elements With Strictly Smaller and Greater Elements - 문제풀이 (0) | 2022.01.23 |
[LeetCode] 2144. Minimum Cost of Buying Candies With Discount - 문제풀이 (0) | 2022.01.23 |