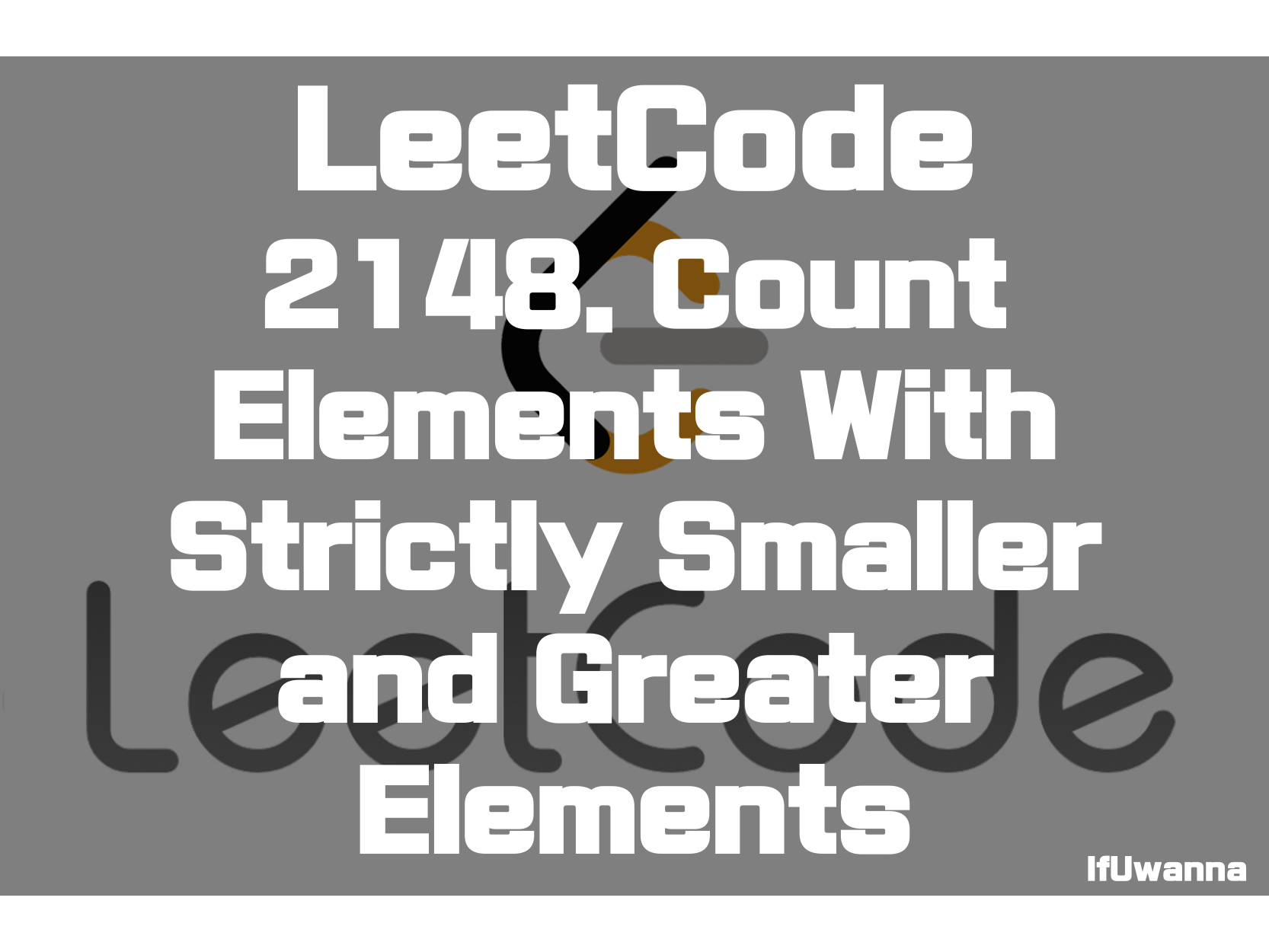
Description
주어진 정수 배열에서, num으로 표시되는 요소 수가 더 적거나 더 많은 모든 요소를 반환합니다. Given an integer array nums, return the number of elements that have both a strictly smaller and a strictly greater element appear in nums.
Example 1:
Input: nums = [11,7,2,15]
Output: 2
Explanation: The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear innums.
Example 2:
Input: nums = [-3,3,3,90]
Output: 2
Explanation: The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear innums.
Constraints:
- 1 <= nums.length <= 100
- 105 <= nums[i] <= 105
Solution 1. Sort
public int countElements(int[] nums) {
int len = nums.length;
Arrays.sort(nums);
int cnt = 0;
int dupCnt = 0;
for (int i = 1; i < len-1; i++) {
if(nums[i] == nums[i+1]){
nums[i] = nums[i-1];
dupCnt++;
}else{
if(nums[i] > nums[i-1] && nums[i] < nums[i+1]){
cnt = cnt + dupCnt + 1;
}
dupCnt = 0;
}
}
return cnt;
}
배열을 정렬 한 뒤 중복값일 경우는 더 작은 값으로 변경해주고 아닐경우 앞뒤 요소를 비교해서 앞으요소보다는 크고 뒤의 요소보다는 작을 경우 cnt를 추가해 반복하며 반환해 줍니다.
Reference
https://leetcode.com/problems/count-elements-with-strictly-smaller-and-greater-elements/
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 387. First Unique Character in a String - 문제풀이 (0) | 2022.01.23 |
---|---|
[LeetCode] 134. Gas Station - 문제풀이 (0) | 2022.01.23 |
[LeetCode] 2144. Minimum Cost of Buying Candies With Discount - 문제풀이 (0) | 2022.01.23 |
[LeetCode] 74. Search a 2D Matrix - 문제풀이 (0) | 2022.01.22 |
[LeetCode] 36. Valid Sudoku - 문제풀이 (0) | 2022.01.22 |