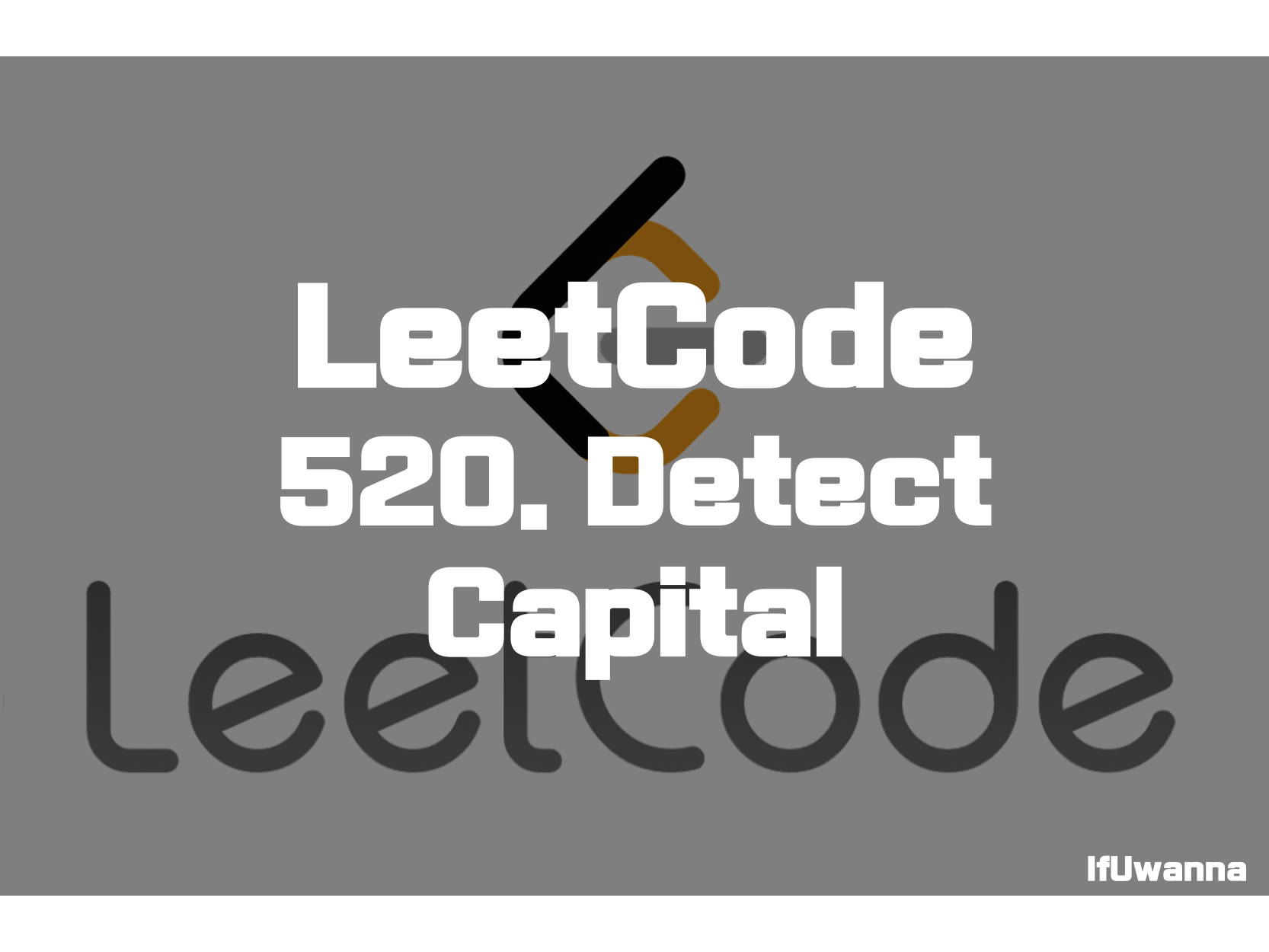
Description
모든문자가 대문자거나, 소문자, 혹은 첫번째 문자만 대문자면서 모든문자가 소문자인 케이스일 경우 true, 이외에 문자는 false를 반환하는 문제입니다.
We define the usage of capitals in a word to be right when one of the following cases holds:
- All letters in this word are capitals, like "USA".
- All letters in this word are not capitals, like "leetcode".
- Only the first letter in this word is capital, like "Google".
Given a string word, return true if the usage of capitals in it is right.
Example 1:
Input: word = "USA"
Output: true
Example 2:
Input: word = "FlaG"
Output: false
Constraints:
- 1 <= word.length <= 100
- word consists of lowercase and uppercase English letters.
Solution 1. Character by Character
public boolean detectCapitalUse(String word) {
// 1. Character by Character
if(word.length() == 1){return true;}
boolean isFirstCapital = word.charAt(0) >= 'A' && word.charAt(0) <= 'Z'? true : false;
boolean isAllCapital = isFirstCapital && word.charAt(1) >= 'A' && word.charAt(1) <= 'Z'? true : false;
for (int i = 1; i < word.length(); i++) {
char c = word.charAt(i);
if (isAllCapital){
if(c < 'A' || c > 'Z') return false; // case1
}else{
if(c < 'a' || c > 'z') return false;// case2.3
}
}
return true;
}
첫번째 두문자로 어떤 케이스에 포함되는지 확인 후 문자를 확인해가며 케이스와 다른 문자가 나올경우 false를 반환하고 모두 통과시 true를 반환합니다.
- 시간 복잡도 : O(N)
- 공간 복잡도 : O(1)
Solution 2. 정규식(Regex)
public boolean detectCapitalUse(String word) {
//2. Regex - 정규식
return word.matches("[A-Z]*|.[a-z]*");
}
정규식을 이용한 방법입니다.
모든 문자가 대문자로 Case1은 정규식 [A-Z]* 로 표현할 수 있고 모든 문자가 소문자인 정규식은 [a-z]로 표현이 가능합니다. 마지막Case3은 [A−Z*][a−z]∗ 로 표현이 가능합니다.
최종적으로 or 연산자를 사용하여 [A-Z]|[a-z]|[A−Z][a−z]∗ 이렇게 표현할 수 있고 case2,3을 묶어서 모든 문자가 올 수 있는 .을 이용하여 [A-Z]*|.[a-z]*로 표현이 가능합니다.
- 시간 복잡도 : O(N)
- 공간 복잡도 : O(1)
Reference
Detect Capital - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 242. Valid Anagram - 문제풀이 (0) | 2022.01.25 |
---|---|
[LeetCode] 941. Valid Mountain Array - 문제풀이 (0) | 2022.01.25 |
[LeetCode] 383. Ransom Note - 문제풀이 (0) | 2022.01.24 |
[LeetCode] 387. First Unique Character in a String - 문제풀이 (0) | 2022.01.23 |
[LeetCode] 134. Gas Station - 문제풀이 (0) | 2022.01.23 |