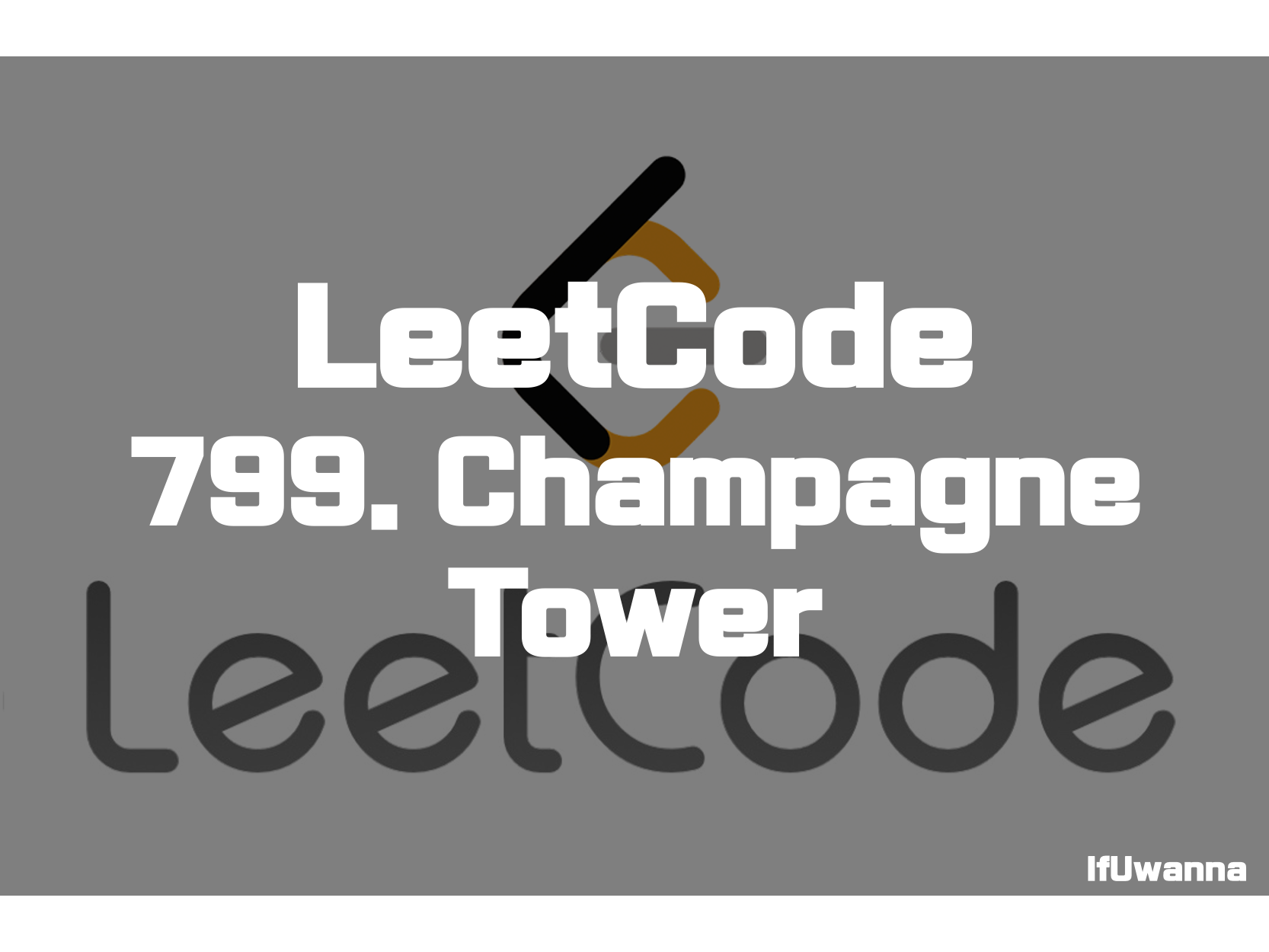
Description
피라미드 형태로 쌓인 컵에 샴페인을 붓는다고 가정할때 주어진 위치의 컵에 담긴 샴페인의 양을 반환하는 문제입니다.
We stack glasses in a pyramid, where the first row has 1 glass, the second row has 2 glasses, and so on until the 100th row. Each glass holds one cup of champagne.
Then, some champagne is poured into the first glass at the top. When the topmost glass is full, any excess liquid poured will fall equally to the glass immediately to the left and right of it. When those glasses become full, any excess champagne will fall equally to the left and right of those glasses, and so on. (A glass at the bottom row has its excess champagne fall on the floor.)
For example, after one cup of champagne is poured, the top most glass is full. After two cups of champagne are poured, the two glasses on the second row are half full. After three cups of champagne are poured, those two cups become full - there are 3 full glasses total now. After four cups of champagne are poured, the third row has the middle glass half full, and the two outside glasses are a quarter full, as pictured below.
Now after pouring some non-negative integer cups of champagne, return how full the jth glass in the ith row is (both i and j are 0-indexed.)
Example 1:
Input: poured = 1, query_row = 1, query_glass = 1
Output: 0.00000
Explanation: We poured 1 cup of champange to the top glass of the tower (which is indexed as (0, 0)). There will be no excess liquid so all the glasses under the top glass will remain empty.
Example 2:
Input: poured = 2, query_row = 1, query_glass = 1
Output: 0.50000
Explanation: We poured 2 cups of champange to the top glass of the tower (which is indexed as (0, 0)). There is one cup of excess liquid. The glass indexed as (1, 0) and the glass indexed as (1, 1) will share the excess liquid equally, and each will get half cup of champange.
Example 3:
Input: poured = 100000009, query_row = 33, query_glass = 17
Output: 1.00000
Constraints:
- 0 <= poured <= 10^9
- 0 <= query_glass <= query_row < 100
Solution 1. Dynamic Programming
public double champagneTower(int poured, int query_row, int query_glass) {
double[][] tower = new double[query_row+1][query_row+1];
tower[0][0] = poured;
for (int r = 0; r < query_row; r++) {
for (int c = 0; c <= r; c++) {
if(tower[r][c] > 1){
double d = (tower[r][c]-1.0) / 2;
tower[r+1][c] += d;
tower[r+1][c+1] += d;
}
}
}
return Math.min(1.0, tower[query_row][query_glass]);
}
동적 프로그래밍 기법을 이용하여 top-down방식으로 계산해 나갑니다. 가장 위에 있는 샴페인에서 컵을 다 채우고 남은양의 절반을 아래에 위치한 컵에 더해 쿼리row 이전까지 계산해 나갑니다.
주어진 컵을 꽉채우고도 남는 케이스를 감안하여 컵에 남아있는 값과 1중에 작은값을 반환해주면 됩니다.
Reference
Champagne Tower - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 15. 3Sum - 문제풀이 (0) | 2022.03.07 |
---|---|
[LeetCode] 740. Delete and Earn - 문제풀이 (0) | 2022.03.05 |
[LeetCode] 413. Arithmetic Slices - 문제풀이 (0) | 2022.03.04 |
[LeetCode] 392. Is Subsequence - 문제풀이 (0) | 2022.03.04 |
[LeetCode] 338. Counting Bits - 문제풀이 (0) | 2022.03.02 |