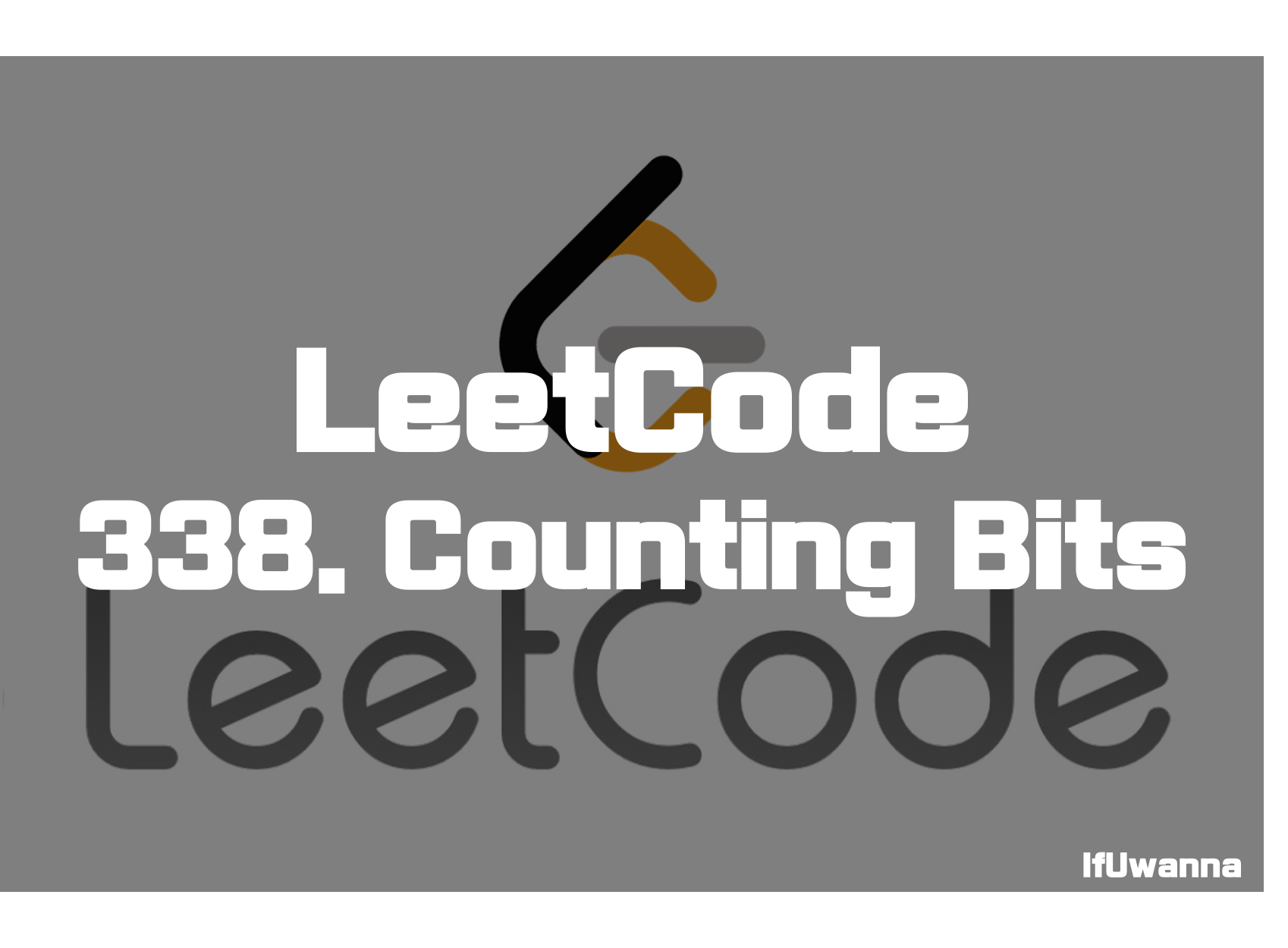
Description
정수가 주어지면 각 ( ) 에 대해 의 이진 표현에서 의 수가 되도록 길이 의 배열n 을 반환 합니다
Given an integer n, return an array ans of length n + 1 such that for each i (0 <= i <= n), ans[i] is the number of 1's* in the binary representation of* i.
Example 1:
Input: n = 2
Output: [0,1,1]
Explanation:
0 --> 0
1 --> 1
2 --> 10
Example 2:
Input: n = 5
Output: [0,1,1,2,1,2]
Explanation:
0 --> 0
1 --> 1
2 --> 10
3 --> 11
4 --> 100
5 --> 101
Constraints:
- 0 <= n <= 10^5
Follow up:
- It is very easy to come up with a solution with a runtime of O(n log n). Can you do it in linear time O(n) and possibly in a single pass?
- Can you do it without using any built-in function (i.e., like __builtin_popcount in C++)?
Solution 1. Bit Manipulation
public int[] countBits(int n) {
int[] result = new int[n+1];
for (int i = 1; i <= n; i++){
result[i] = result[i >> 1] + (i & 1);
}
return result;
}
Solution 2. bitCount()
public int[] countBits(int n) {
int[] result = new int[n+1];
for(int i = 0; i<=n; i++){
result[i] = (Integer.bitCount(i));
}
return result;
}
Reference
Counting Bits - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 413. Arithmetic Slices - 문제풀이 (0) | 2022.03.04 |
---|---|
[LeetCode] 392. Is Subsequence - 문제풀이 (0) | 2022.03.04 |
[LeetCode] 228. Summary Ranges - 문제풀이 (0) | 2022.02.28 |
[LeetCode] 165. Compare Version Numbers - 문제풀이 (0) | 2022.02.25 |
[LeetCode] 148. Sort List - 문제풀이 (0) | 2022.02.24 |