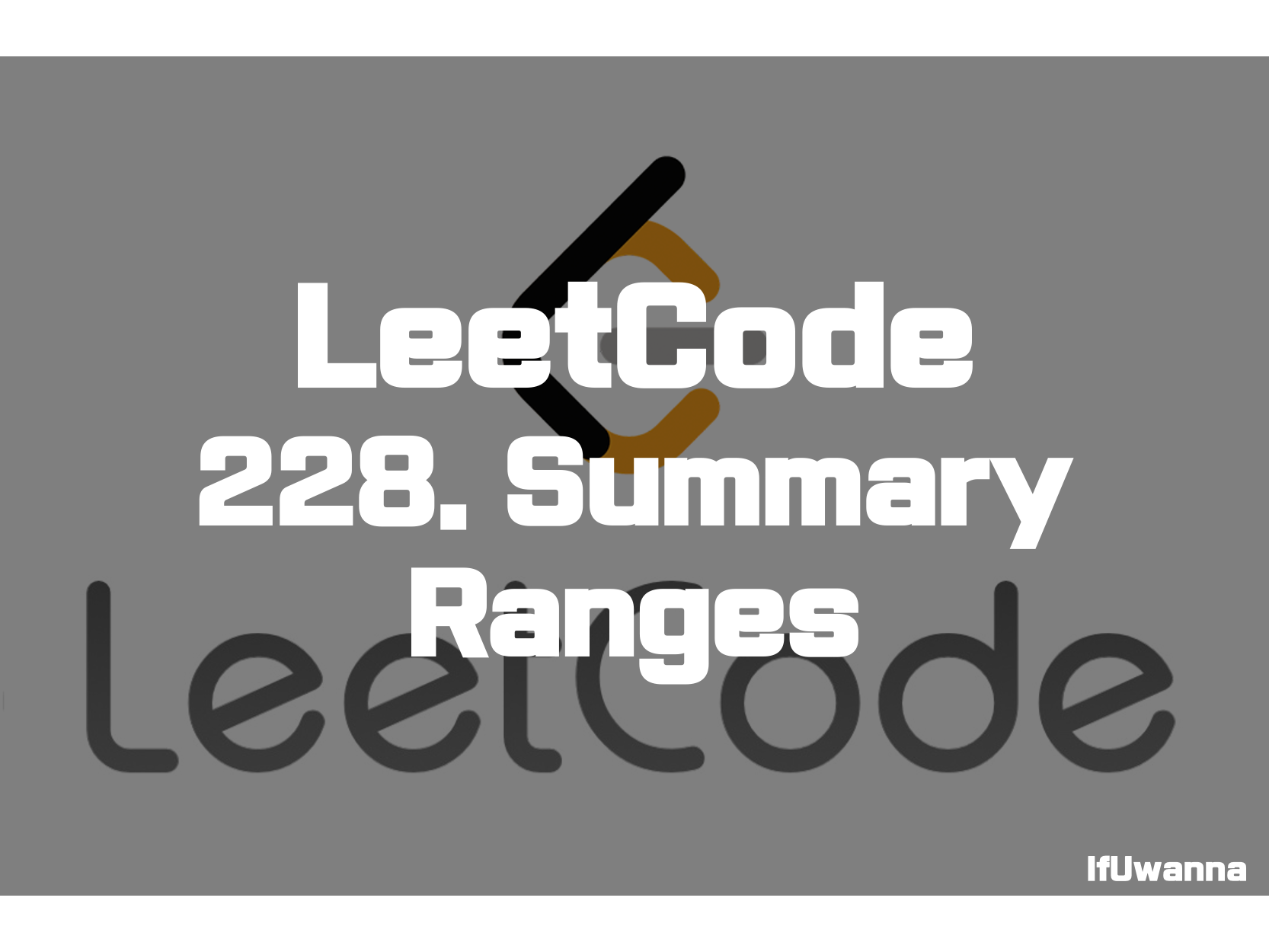
Description
정렬된 유일한 값을 가지는 배열이 주어졌을때 배열의 모든 숫자를 정확히 포함 하는 가장 작은 정렬된 범위 목록을 반환 합니다 .
You are given a sorted unique integer array nums.
Return the smallest sorted list of ranges that cover all the numbers in the array exactly. That is, each element of nums is covered by exactly one of the ranges, and there is no integer x such that x is in one of the ranges but not in nums.
Each range [a,b] in the list should be output as:
- "a->b" if a != b
- "a" if a == b
Example 1:
Input: nums = [0,1,2,4,5,7]
Output: ["0->2","4->5","7"]
Explanation: The ranges are:
[0,2] --> "0->2"
[4,5] --> "4->5"
[7,7] --> "7"
Example 2:
Input: nums = [0,2,3,4,6,8,9]
Output: ["0","2->4","6","8->9"]
Explanation: The ranges are:
[0,0] --> "0"
[2,4] --> "2->4"
[6,6] --> "6"
[8,9] --> "8->9"
Constraints:
- 0 <= nums.length <= 20
- 2^31 <= nums[i] <= 2^31 - 1
- All the values of nums are unique.
- nums is sorted in ascending order.
Solution 1. 반복
public List<String> summaryRanges(int[] nums) {
List<String> result = new ArrayList<>();
int len = nums.length;
if(len == 0){
return result;
}
int start = nums[0];
int end = nums[0];
for (int i = 0; i < len; i++) {
end = nums[i];
if( i == len-1 || nums[i]+1 != nums[i+1] ) {
if (start == end) {
result.add(String.valueOf(start));
} else {
result.add(start + "->" + end);
}
if(i != len-1)start = nums[i+1];
}
}
return result;
}
시작점과 끝점을 기록하며 반복하며 다음요소와 현재 요소가 끊어졌을때(nums[i]+1 != nums[i+1]) 결과값을 기록합니다.
Reference
Summary Ranges - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 392. Is Subsequence - 문제풀이 (0) | 2022.03.04 |
---|---|
[LeetCode] 338. Counting Bits - 문제풀이 (0) | 2022.03.02 |
[LeetCode] 165. Compare Version Numbers - 문제풀이 (0) | 2022.02.25 |
[LeetCode] 148. Sort List - 문제풀이 (0) | 2022.02.24 |
[LeetCode] 133. Clone Graph - 문제풀이 (0) | 2022.02.23 |