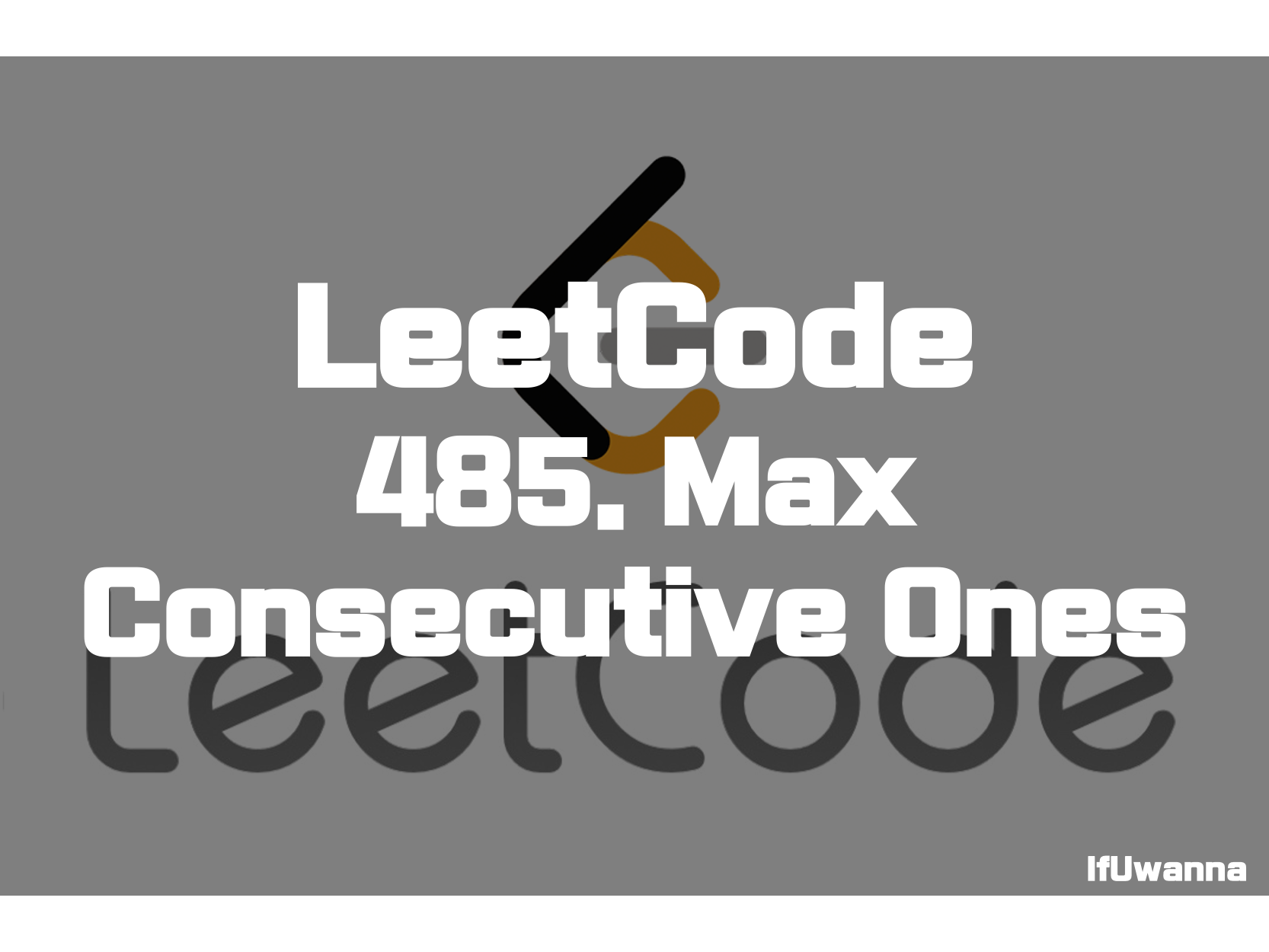
Description
주어진 배열에서 최대로 연속되는 1의 개수를 구하는 문제입니다.
Given a binary array nums, return the maximum number of consecutive 1's in the array.
Example 1:
Input: nums = [1,1,0,1,1,1]
Output: 3
Explanation: The first two digits or the last three digits are consecutive 1s. The maximum number of consecutive 1s is 3.
Example 2:
Input: nums = [1,0,1,1,0,1]
Output: 2
Constraints:
- 1 <= nums.length <= 10^5
- nums[i] is either 0 or 1.
Solution 1. Arrays
public int findMaxConsecutiveOnes(int[] nums) {
int len = nums.length;
int max = 0;
int sum = 0;
for(int i = 0; i < len; i++){
if(nums[i] != 0){
sum += nums[i];
}else{ // zero case
max = Math.max(sum,max);
sum = 0;
}
}
return Math.max(sum,max);
}
배열을 순회하며 1일 경우 count를 더하고 0이 나오면 현재까지의 sum과 max를 비교하여 큰값을 기록한 뒤 max값을 반환해줍니다.
Reference
Max Consecutive Ones - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 402. Remove K Digits - 문제풀이 (0) | 2022.02.18 |
---|---|
[LeetCode] 39. Combination Sum - 문제풀이 (0) | 2022.02.17 |
[LeetCode] 24. Swap Nodes in Pairs - 문제풀이 (0) | 2022.02.16 |
[LeetCode] 136. Single Number - 문제풀이 (0) | 2022.02.15 |
[LeetCode] 190. Reverse Bits - 문제풀이 (0) | 2022.02.15 |