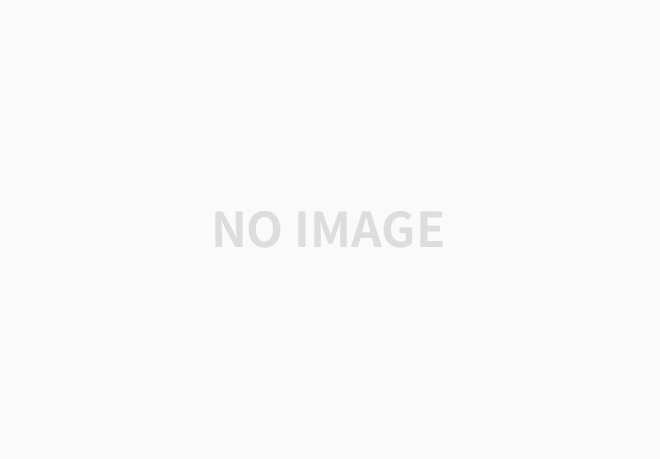
Description
주어진 부호없는 32비트 정수를 뒤집어서 반환하는 문제입니다.
Reverse bits of a given 32 bits unsigned integer.
Note:
- Note that in some languages, such as Java, there is no unsigned integer type. In this case, both input and output will be given as a signed integer type. They should not affect your implementation, as the integer's internal binary representation is the same, whether it is signed or unsigned.
- In Java, the compiler represents the signed integers using 2's complement notation. Therefore, in Example 2 above, the input represents the signed integer 3 and the output represents the signed integer 1073741825.
Example 1:
Input: n = 00000010100101000001111010011100
Output: 964176192 (00111001011110000010100101000000)
Explanation:The input binary string00000010100101000001111010011100 represents the unsigned integer 43261596, so return 964176192 which its binary representation is00111001011110000010100101000000.
Example 2:
Input: n = 11111111111111111111111111111101
Output: 3221225471 (10111111111111111111111111111111)
Explanation:The input binary string11111111111111111111111111111101 represents the unsigned integer 4294967293, so return 3221225471 which its binary representation is10111111111111111111111111111111.
Constraints:
- The input must be a binary string of length 32
Follow up: If this function is called many times, how would you optimize it?
Solution 1. bit Shift
public int reverseBits(int n) {
int result = 0;
for (int i = 0; i < 32; i++) {
result += n & 1;
n >>>= 1; // must do unsigned shift
if (i < 31) // for last digit, don't shift!
result <<= 1;
}
return result;
}
result(0 000000)에 기존 값 n의 끝자리를 &1로 추출하여 제일 오른쪽에 더해주고 기존값은 부호없이 오른쪽으로 shift(>>>) 결과는 왼쪽으로 shift하여 역순으로 bit를 나열해줍니다.
Reference
Reverse Bits - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com