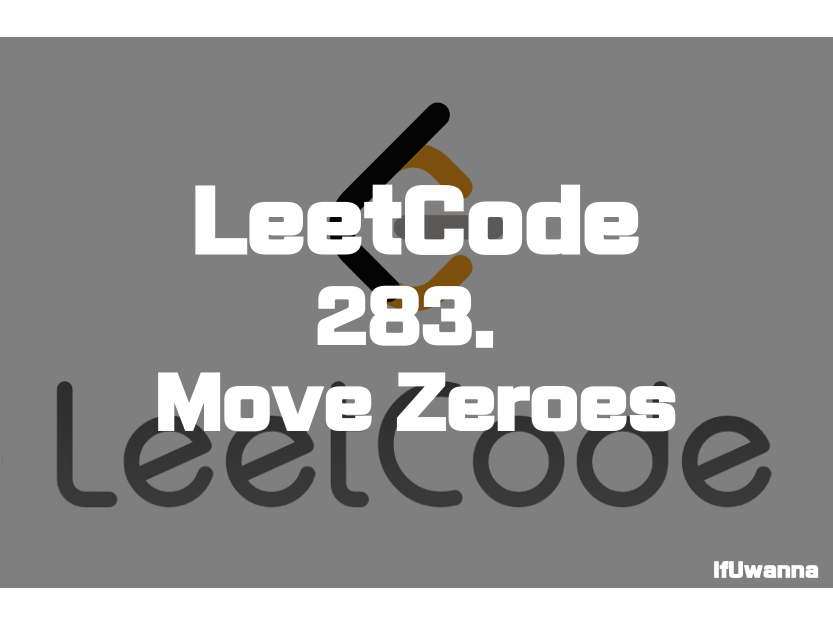
Description
주어진 배열내의 모든 0값을 가진 요소를 제일 오른쪽으로 이동시키는 문제입니다.
Given an integer array nums, move all 0's to the end of it while maintaining the relative order of the non-zero elements.
Note that you must do this in-place without making a copy of the array.
Example 1:
Input: nums = [0,1,0,3,12]
Output: [1,3,12,0,0]
Example 2:
Input: nums = [0]
Output: [0]
Constraints:
- 1 <= nums.length <= 10^4
- -2^31 <= nums[i] <= 2^31 - 1
Follow up:
Could you minimize the total number of operations done?
Solution 1.
public void moveZeroes(int[] nums) {
// 1. Using Pointer
int len = nums.length;
int idx = 0;
for(int i=0; i<len; i++){
if(nums[i] != 0){
nums[idx++] = nums[i];
}
}
Arrays.fill(nums,idx,len,0);
}
배열을 순회하며 0이 아닐경우 인덱스(idx) 0부터 채워가며 이동시킵니다.
Reference
Move Zeroes - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 344. Reverse String - 문제풀이 (0) | 2022.01.20 |
---|---|
[LeetCode] 167. Two Sum II - Input Array Is Sorted - 문제풀이 (0) | 2022.01.20 |
[LeetCode] 142. Linked List Cycle II - 문제 풀이 (0) | 2022.01.20 |
[LeetCode] 605. Can Place Flowers - 문제풀이 (0) | 2022.01.18 |
[LeetCode] 88. Merge Sorted Array - 문제풀이 (0) | 2022.01.18 |