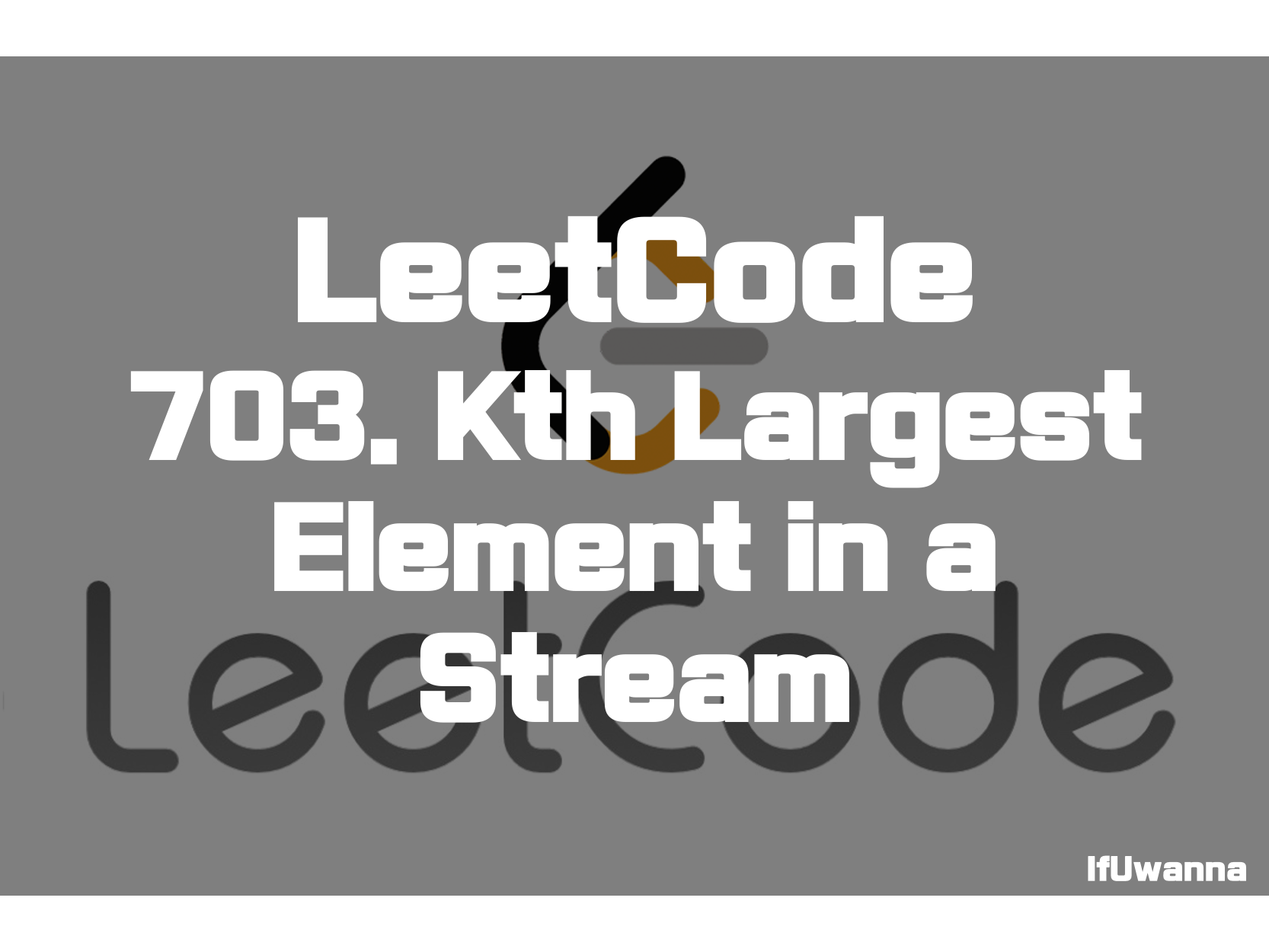
Description
스트림에 정렬된 순서에서 가장 큰 요소를 찾는 클래스를 디자인 합니다.
Design a class to find the kth largest element in a stream. Note that it is the kth largest element in the sorted order, not the kth distinct element.
Implement KthLargest class:
- KthLargest(int k, int[] nums) Initializes the object with the integer k and the stream of integers nums.
- int add(int val) Appends the integer val to the stream and returns the element representing the kth largest element in the stream.
Example 1:
Input
["KthLargest", "add", "add", "add", "add", "add"]
[[3, [4, 5, 8, 2]], [3], [5], [10], [9], [4]]
Output
[null, 4, 5, 5, 8, 8]
Explanation
KthLargest kthLargest = new KthLargest(3, [4, 5, 8, 2]);
kthLargest.add(3); // return 4
kthLargest.add(5); // return 5
kthLargest.add(10); // return 5
kthLargest.add(9); // return 8
kthLargest.add(4); // return 8
Constraints:
- 1 <= k <= 104
- 0 <= nums.length <= 104
- -10^4 <= nums[i] <= 10^4
- -10^4 <= val <= 10^4
- At most 10^4 calls will be made to add.
- It is guaranteed that there will be at least k elements in the array when you search for the kth element.
Solution 1. PriorityQueue
public class KthLargest {
private final PriorityQueue<Integer> q;
private final int k;
public KthLargest(int k, int[] nums) {
this.k = k;
q = new PriorityQueue<Integer>(k);
for (int a :nums) {
add(a);
}
}
public int add(int val) {
q.offer(val);
if (q.size() > k) {
q.poll();
}
return q.peek();
}
}
우선순위 큐를 이용하여 k사이즈보다 큰 값은 poll해가며 우선순위를 유지한뒤 가장 큰값을 peek 해줍니다.
Reference
Kth Largest Element in a Stream - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 905. Sort Array By Parity - 문제풀이 (0) | 2022.05.02 |
---|---|
[LeetCode] 669. Trim a Binary Search Tree - 문제풀이 (0) | 2022.04.26 |
[LeetCode] 1046. Last Stone Weight - 문제풀이 (0) | 2022.04.07 |
[LeetCode] 923. 3Sum With Multiplicity - 문제풀이 (0) | 2022.04.06 |
[LeetCode] 11. Container With Most Water - 문제풀이 (0) | 2022.04.06 |