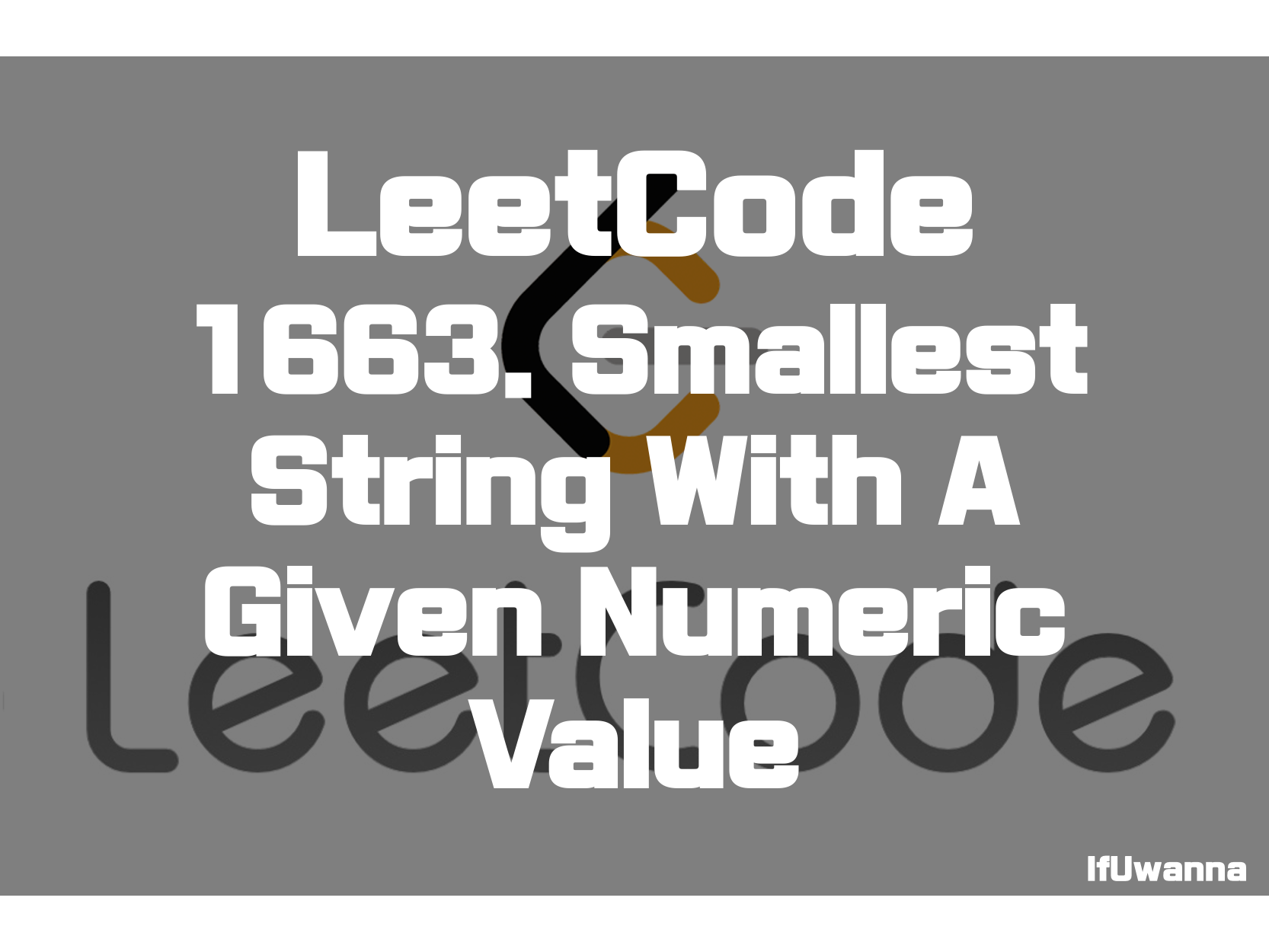
Description
a~z(1~26)까지라고 할때 n개의 알파벳으로 k만큽 합계를 나타낼 수 사전순으로 가장 작은 문자열의 알파벳을 반환하는 문제입니다.
The numeric value of a lowercase character is defined as its position (1-indexed) in the alphabet, so the numeric value of a is 1, the numeric value of b is 2, the numeric value of c is 3, and so on.
The numeric value of a string consisting of lowercase characters is defined as the sum of its characters' numeric values. For example, the numeric value of the string "abe" is equal to 1 + 2 + 5 = 8.
You are given two integers n and k. Return the lexicographically smallest string with length equal to n and numeric value equal to k.
Note that a string x is lexicographically smaller than string y if x comes before y in dictionary order, that is, either x is a prefix of y, or if i is the first position such that x[i] != y[i], then x[i] comes before y[i] in alphabetic order.
Example 1:
Input: n = 3, k = 27
Output: "aay"
Explanation: The numeric value of the string is 1 + 1 + 25 = 27, and it is the smallest string with such a value and length equal to 3.
Example 2:
Input: n = 5, k = 73
Output: "aaszz"
Constraints:
- 1 <= n <= 10^5
- n <= k <= 26 * n
Solution 1. Greedy
public String getSmallestString(int n, int k) {
char res[] = new char[n];
Arrays.fill(res, 'a');
k -= n;
while(k > 0){
res[--n] += Math.min(25, k);
k -= Math.min(25, k);
}
return String.valueOf(res);
}
결과 배열을 개수를 모두 a로 채운뒤 개수에 맞게 k값을 1씩 제외해 줍니다. 그후 배열의 맨 뒤부터 스캔하며 k가 26 보다 많이 남아있으면 25를 더해 z로 만들어 주며 k가 0이 될때까지 반복합니다.
Reference
Smallest String With A Given Numeric Value - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 1337. The K Weakest Rows in a Matrix - 문제풀이 (0) | 2022.03.27 |
---|---|
[LeetCode] 1029. Two City Scheduling - 문제풀이 (0) | 2022.03.25 |
[LeetCode] 991. Broken Calculator - 문제풀이 (0) | 2022.03.25 |
[LeetCode] 881. Boats to Save People - 문제풀이 (0) | 2022.03.25 |
[LeetCode] 763. Partition Labels - 문제풀이 (0) | 2022.03.21 |