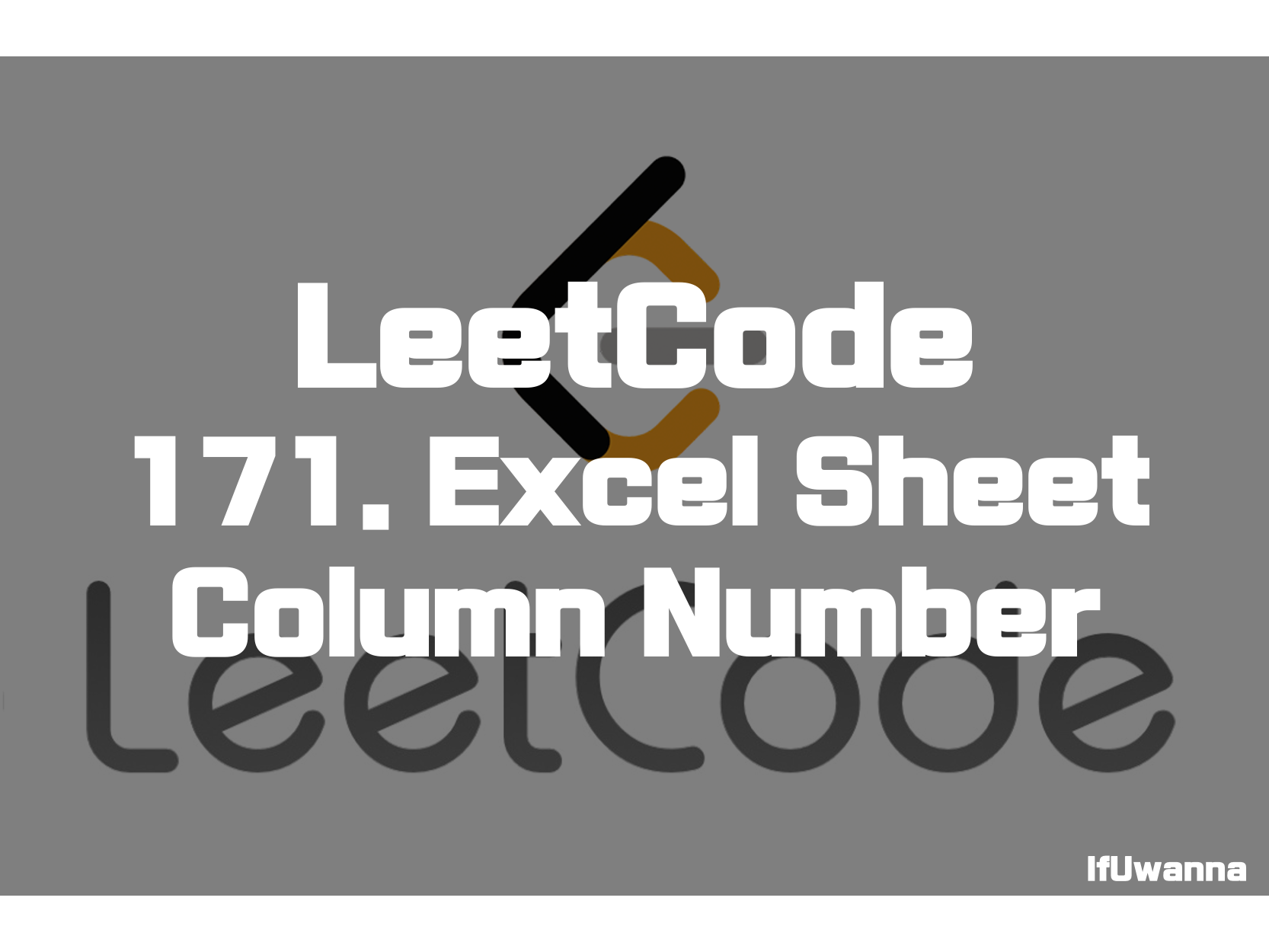
Description
Excel 시트에 나타나는 열 제목을 나타내는 문자열 columnTitle이 지정된 경우 해당 열 번호를 반환합니다.
Given a string columnTitle that represents the column title as appear in an Excel sheet, return its corresponding column number.
For example:
A -> 1
B -> 2
C -> 3
...
Z -> 26
AA -> 27
AB -> 28
...
Example 1:
Input: columnTitle = "A"
Output: 1
Example 2:
Input: columnTitle = "AB"
Output: 28
Example 3:
Input: columnTitle = "ZY"
Output: 701
Constraints:
- 1 <= columnTitle.length <= 7
- columnTitle consists only of uppercase English letters.
- columnTitle is in the range ["A", "FXSHRXW"].
Solution 1. Math
public int titleToNumber(String columnTitle) {
int result = 0;
int len = columnTitle.length();
for (int i = 0; i < len; i++) {
int idx = len-1-i;
result += Math.pow(26,i)*(columnTitle.charAt(idx)-'A'+1);
}
return result;
}
알파벳은 26개씩 반복되므로 알파벳으로 구성된 26진수라고 생각하고 끝자리부터 Math.pow 함수를 이용해 26의 0,1,2.. 제곱에 각 알파벳을 곱한 합을 반환해줍니다.
Reference
Excel Sheet Column Number - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 148. Sort List - 문제풀이 (0) | 2022.02.24 |
---|---|
[LeetCode] 133. Clone Graph - 문제풀이 (0) | 2022.02.23 |
[LeetCode] 169. Majority Element - 문제풀이 (0) | 2022.02.21 |
[LeetCode] 1288. Remove Covered Intervals - 문제풀이 (0) | 2022.02.20 |
[LeetCode] 402. Remove K Digits - 문제풀이 (0) | 2022.02.18 |