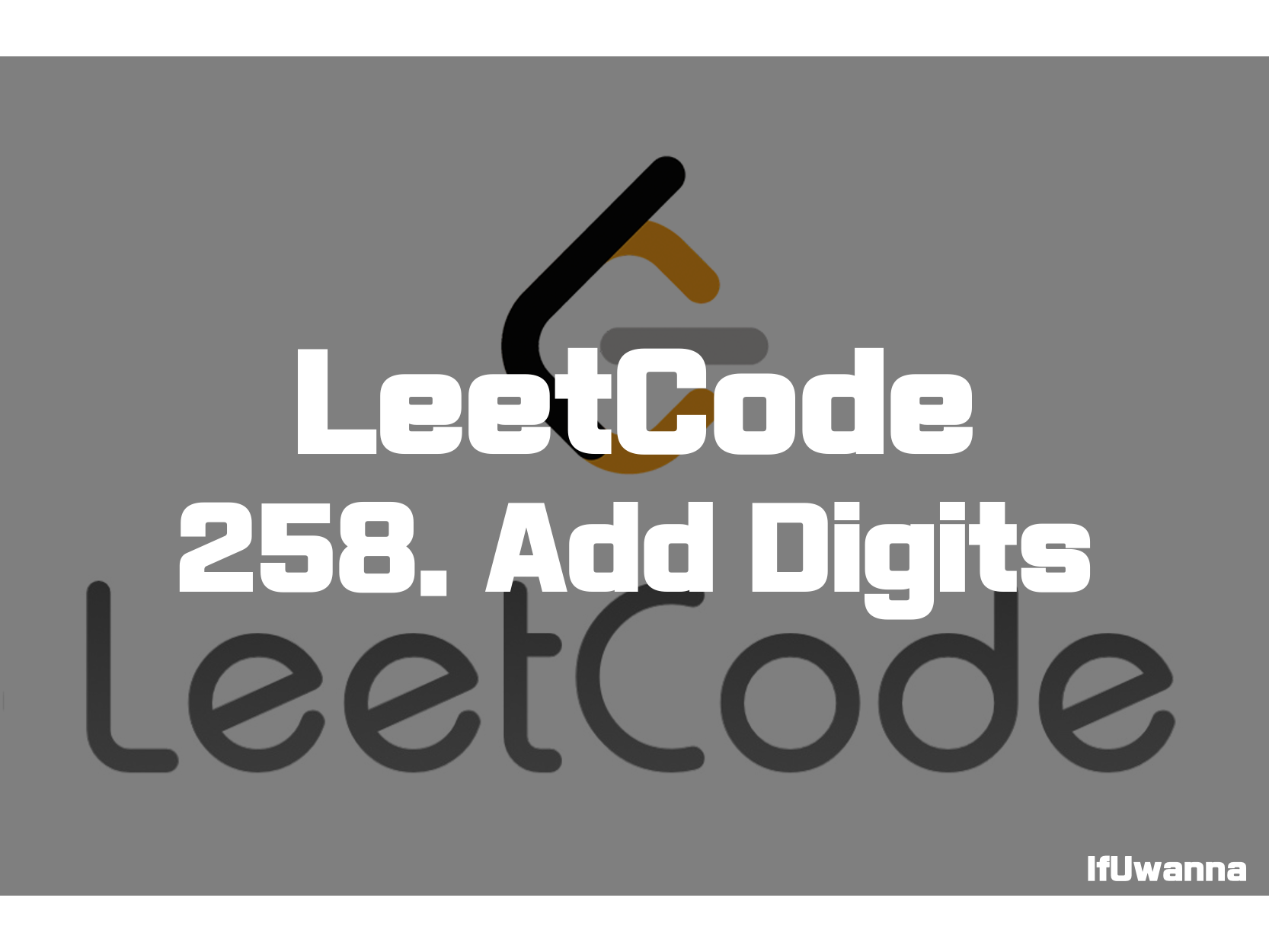
Description
정수num이 주어졌을때 각 자리별로 한자리 수가 될때까지 더하여 반환하는 문제입니다.
Given an integer num, repeatedly add all its digits until the result has only one digit, and return it.
Example 1:
Input: num = 38
Output: 2
Explanation: The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
Example 2:
Input: num = 0
Output: 0
Constraints:
- 0 <= num <= 2^31 - 1
Follow up: Could you do it without any loop/recursion in O(1) runtime?
Solution 1. Math
public int addDigits(int num) {
//1. Math
if (num == 0) return 0;
if (num % 9 == 0) return 9;
return num % 9;
}
아래 수학 공식을 통하여 결과를 도출합니다.
Solution 2. 반복
public int addDigits(int num) {
//2. iteration
String s = String.valueOf(num);
while(true){
int sum = 0;
for(char c : s.toCharArray()){
int i = c-'0';
sum += i;
}
if(sum/10 == 0){
return sum;
}
s = String.valueOf(sum);
}
}
반복문을 이용하여 각 자리수별로 더하여 한자리가 될 때 결과를 반환합니다.
Reference
Add Digits - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 560. Subarray Sum Equals K - 문제풀이 (0) | 2022.02.11 |
---|---|
[LeetCode] 532. K-diff Pairs in an Array - 문제풀이 (0) | 2022.02.09 |
[LeetCode] 389. Find the Difference - 문제풀이 (0) | 2022.02.07 |
[LeetCode] 102. Binary Tree Level Order Traversal - 문제풀이 (0) | 2022.02.06 |
[LeetCode] 80. Remove Duplicates from Sorted Array II - 문제풀이 (0) | 2022.02.06 |