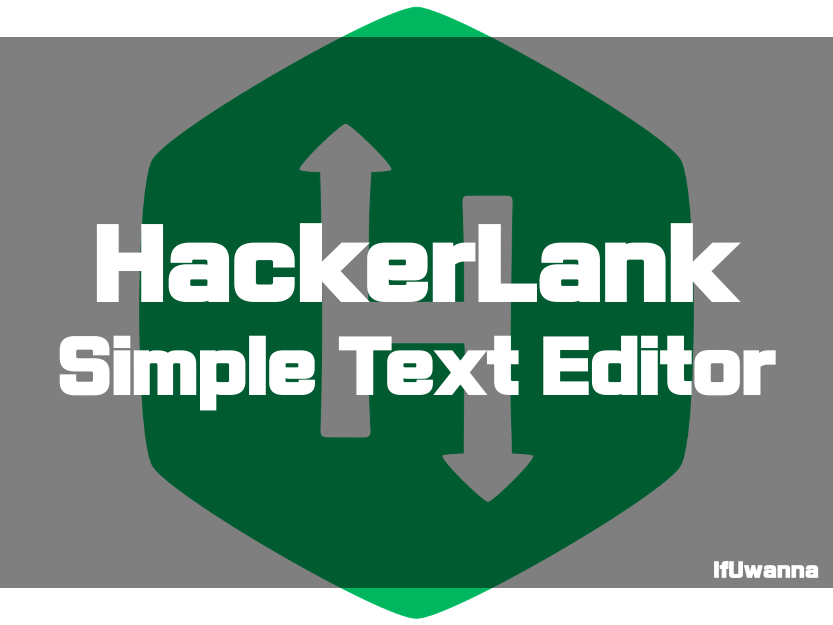
Description
주어진 스펙을 가지는 간단한 텍스트 에디터를 구현하는 문제입니다.
Solution 1. Stack
public static void main(String[] args) {
TextEditor editor = new TextEditor("");
Scanner in = new Scanner(System.in);
while(in.hasNext()){
int cm = in.nextInt();
switch (cm){
case 1 : editor.append(in.next()); break;
case 2 : editor.delete(in.nextInt()); break;
case 3 : editor.print(in.nextInt()); break;
case 4 : editor.undo(); break;
default: break;
}
}
}
public static class TextEditor<Integer> {
private Stack<String> stack = new Stack();
public TextEditor(String text) {
stack.push(text);
}
public void append(String s){
stack.push(stack.peek() + s);
}
public void delete(int n){
String s = stack.peek();
if(s.length() < n){
stack.push("");
}else{
stack.push(s.substring(0,s.length()-n));
}
}
public void print(int i){
System.out.println(stack.peek().charAt(i-1));
}
public void undo(){
if(!stack.isEmpty()){
stack.pop();
}
}
}
스택을 이용하여 이전 기록들을 관리하며 값을 변경해 나갑니다.
Reference
Simple Text Editor | HackerRank
Implement a simple text editor and perform some number of operations.
www.hackerrank.com
'알고리즘 > HackerRank' 카테고리의 다른 글
[HakcerLank] Pairs - 문제풀이 (0) | 2022.03.25 |
---|---|
[HackerLank] Balanced Brackets - 문제풀이 (0) | 2022.03.25 |
[HackerLank] Queue using Two Stacks - 문제풀이 (0) | 2022.03.25 |
[HackerLank] Merge two sorted linked lists - 문제풀이 (0) | 2022.03.25 |
[HackerLank] Truck Tour - 문제풀이 (0) | 2022.03.25 |